定义一个抽象类AbsAnimal,要求如下 (1)包括属性name,weight,color; (2)定义其有参构造方法; (3)定义受保护的run方法,功能是打印输出"Animal run fastly"; (4)在main方法里实例化抽象对象a AbsAnimal a = new AbsAnimal(….) ,想一想为什么会报错?如果报错注释掉该语句。
时间: 2023-12-06 11:44:14 浏览: 63
以下是抽象类AbsAnimal的代码实现:
```java
public abstract class AbsAnimal {
protected String name;
protected double weight;
protected String color;
public AbsAnimal(String name, double weight, String color) {
this.name = name;
this.weight = weight;
this.color = color;
}
protected void run() {
System.out.println("Animal run fastly");
}
}
```
在main方法里实例化抽象对象a AbsAnimal a = new AbsAnimal(….),会报错,因为抽象类无法被实例化。如果想要创建AbsAnimal对象,需要定义一个非抽象的子类实现AbsAnimal,并在子类中重写AbsAnimal中的抽象方法,之后再实例化子类对象。
相关问题
java定义一个抽象类AbsAnimal,要求如下 (1)包括属性name,weight,color; (2)定义其有参构造方法 (3)定义受保护的run方法,功能是打印输出"Animal run fastly" 定义一个类Tiger继承抽象类AbsAnimal, 要求如下(1)在main方法中实例化一个Tiger对象(2)调用run方法,查看输出结果
好的,这是Java定义抽象类AbsAnimal并继承的类Tiger的代码:
```java
public abstract class AbsAnimal {
protected String name;
protected double weight;
protected String color;
public AbsAnimal(String name, double weight, String color) {
this.name = name;
this.weight = weight;
this.color = color;
}
protected void run() {
System.out.println("Animal run fastly");
}
}
public class Tiger extends AbsAnimal {
public Tiger(String name, double weight, String color) {
super(name, weight, color);
}
}
public class Main {
public static void main(String[] args) {
Tiger tiger = new Tiger("Tiger", 200.0, "Orange");
tiger.run();
}
}
```
在这个代码中,我们定义了一个抽象类AbsAnimal,并在其子类Tiger中实现了具体的构造方法。在main方法中,我们实例化了一个Tiger对象,并调用其run方法,输出结果为"Animal run fastly"。
需要注意的是,由于AbsAnimal是一个抽象类,我们无法直接实例化它,只能通过其具体的子类来实例化。同时,由于run方法是受保护的,我们只能在其子类中调用该方法。
定义一个抽象类AbsAnimal,要求如下 (1)包括属性name,weight,color; (2)定义其有参构造方法; (3)定义受保护的run方法,功能是打印输出"Animal run fastly"; (4)在main方法里实例化抽象对象a 定义一个类Tiger继承抽象类AbsAnimal,要求如下 (1)在main方法中实例化一个Tiger对象 (2)调用run方法,查看输出结果
好的,我来解答您的问题。
下面是定义一个抽象类 `AbsAnimal` 的代码:
```java
public abstract class AbsAnimal {
protected String name;
protected double weight;
protected String color;
public AbsAnimal(String name, double weight, String color) {
this.name = name;
this.weight = weight;
this.color = color;
}
protected void run() {
System.out.println("Animal run fastly");
}
}
```
接下来是定义一个继承自 `AbsAnimal` 的 `Tiger` 类的代码:
```java
public class Tiger extends AbsAnimal {
public Tiger(String name, double weight, String color) {
super(name, weight, color);
}
public static void main(String[] args) {
Tiger tiger = new Tiger("Tiger", 100, "Orange");
tiger.run();
}
}
```
在 `Tiger` 类的 `main` 方法中,我们实例化了一个 `Tiger` 对象,并调用了其继承自 `AbsAnimal` 的 `run` 方法,输出了 "Animal run fastly"。
需要注意的是,由于 `AbsAnimal` 类是一个抽象类,不能直接实例化对象,因此我们只能通过继承 `AbsAnimal` 类的子类来实例化对象。
阅读全文
相关推荐
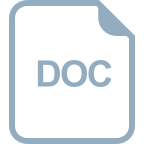
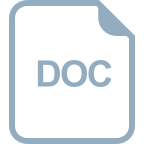
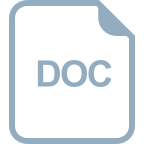









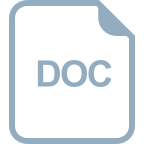
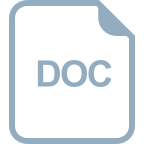
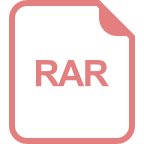
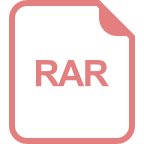