Qt绘制多个ROI区域并可选择ROI区域
时间: 2024-03-29 15:34:11 浏览: 31
您好!感谢您的提问。在Qt中,可以使用QGraphicsView和QGraphicsScene来实现绘制多个ROI区域并可选择ROI区域的功能。
首先,您需要创建一个QGraphicsScene对象,并将其设置为QGraphicsView的场景。然后,您可以创建一个自定义的图形项类,并在其中实现绘制ROI区域的功能。在图形项类中,您可以重写mousePressEvent和mouseReleaseEvent等事件函数,以实现选择ROI区域的功能。
以下是一个简单的示例代码,用于绘制矩形ROI区域并选择ROI区域:
```
#include <QtWidgets>
class ROIItem : public QGraphicsItem {
public:
ROIItem(QGraphicsItem *parent = nullptr) : QGraphicsItem(parent) {
setFlags(ItemIsSelectable | ItemIsMovable);
}
QRectF boundingRect() const override {
return QRectF(0, 0, m_width, m_height);
}
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget = nullptr) override {
painter->drawRect(0, 0, m_width, m_height);
}
void setRect(const QRectF &rect) {
prepareGeometryChange();
m_width = rect.width();
m_height = rect.height();
}
private:
qreal m_width = 0;
qreal m_height = 0;
};
class GraphicsScene : public QGraphicsScene {
public:
GraphicsScene(QObject *parent = nullptr) : QGraphicsScene(parent) {}
void mousePressEvent(QGraphicsSceneMouseEvent *event) override {
if (event->button() == Qt::LeftButton) {
QPointF pos = event->scenePos();
ROIItem *item = new ROIItem();
item->setRect(QRectF(pos, QSizeF(0, 0)));
addItem(item);
m_currentItem = item;
}
QGraphicsScene::mousePressEvent(event);
}
void mouseMoveEvent(QGraphicsSceneMouseEvent *event) override {
if (m_currentItem) {
QPointF pos = event->scenePos();
qreal width = pos.x() - m_currentItem->scenePos().x();
qreal height = pos.y() - m_currentItem->scenePos().y();
m_currentItem->setRect(QRectF(0, 0, width, height));
}
QGraphicsScene::mouseMoveEvent(event);
}
void mouseReleaseEvent(QGraphicsSceneMouseEvent *event) override {
m_currentItem = nullptr;
QGraphicsScene::mouseReleaseEvent(event);
}
private:
ROIItem *m_currentItem = nullptr;
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QGraphicsView view;
GraphicsScene scene;
view.setScene(&scene);
view.show();
return app.exec();
}
```
在这个示例中,我们创建了一个ROIItem类,并实现了paint函数来绘制矩形ROI区域。在GraphicsScene类中,我们重写了mousePressEvent、mouseMoveEvent和mouseReleaseEvent等事件函数,并在其中创建、绘制和选择ROI区域。最后,我们创建了一个QGraphicsView对象,并将其设置为GraphicsScene的场景,以显示ROI区域。
希望这个示例能够帮助您实现绘制多个ROI区域并可选择ROI区域的功能。如果您还有任何其他问题,请随时问我。
相关推荐
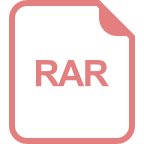
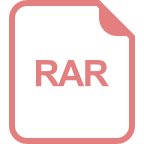













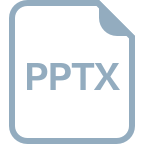
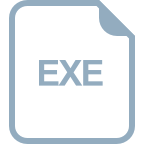