配置USART3的参数 使用MDB协议
时间: 2024-06-12 12:04:31 浏览: 130
以下是配置USART3的参数,使用MDB协议的示例代码:
```c
#include "stm32f4xx.h"
void init_USART3(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
USART_InitTypeDef USART_InitStruct;
NVIC_InitTypeDef NVIC_InitStruct;
// Enable the clock for the USART3 peripheral and GPIOB
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART3, ENABLE);
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
// Configure the GPIO pins for USART3
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_10 | GPIO_Pin_11;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOB, &GPIO_InitStruct);
// Connect the USART3 pins to the alternate function
GPIO_PinAFConfig(GPIOB, GPIO_PinSource10, GPIO_AF_USART3); // PB10 -> Tx
GPIO_PinAFConfig(GPIOB, GPIO_PinSource11, GPIO_AF_USART3); // PB11 -> Rx
// Configure the USART3 parameters
USART_InitStruct.USART_BaudRate = 115200;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART3, &USART_InitStruct);
// Enable the USART3 receive interrupt
USART_ITConfig(USART3, USART_IT_RXNE, ENABLE);
// Configure the NVIC for USART3
NVIC_InitStruct.NVIC_IRQChannel = USART3_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
// Enable the USART3 peripheral
USART_Cmd(USART3, ENABLE);
}
void USART3_IRQHandler(void)
{
if (USART_GetITStatus(USART3, USART_IT_RXNE) != RESET)
{
// Read the received byte from USART3
uint8_t data = USART_ReceiveData(USART3);
// Process the received byte using MDB protocol
// ...
// Clear the USART3 receive interrupt flag
USART_ClearITPendingBit(USART3, USART_IT_RXNE);
}
}
```
在`init_USART3()`函数中,我们首先启用了USART3和GPIOB的时钟,并针对GPIOB的PB10和PB11两个引脚配置了GPIO初始化结构体`GPIO_InitStruct`,然后将其连接到USART3的备用功能上,并配置了USART3的初始化结构体`USART_InitStruct`,包括波特率、数据位、停止位、校验位、硬件流控制和模式等。在此之后,我们还启用了USART3的接收中断,并为USART3配置了NVIC中断向量,最后启用了USART3外设。
在`USART3_IRQHandler()`中断处理函数中,我们检查USART3是否接收到了数据,并读取该数据。接下来,我们使用MDB协议处理接收到的字节,该协议用于连接售货机的外设,如硬币器和纸币器等。最后,我们清除了USART3的接收中断标志位。
阅读全文
相关推荐
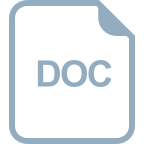
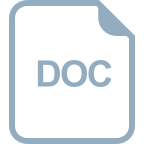
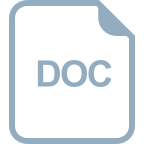



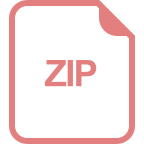
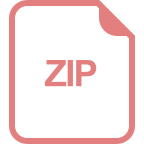
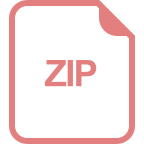
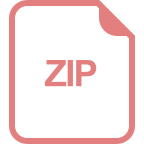
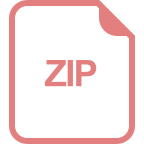
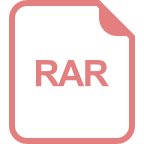
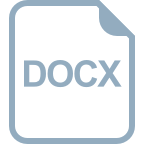
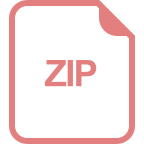
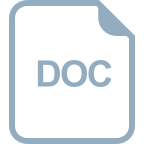
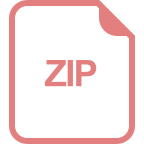
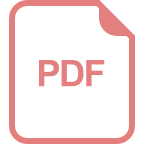