设A、B是两个整数集合,每个集合中的元素数均在100以内。这里要求编写一个函数来实现A∪B的集合运算,运算结果存放在集合C中(集合C中的元素有可能突破100).c语言解题
时间: 2023-11-05 14:05:15 浏览: 124
可以使用数组来表示集合,数组下标表示集合中的元素,数组元素为1表示该元素在集合中,为0表示不在集合中。可以按照如下步骤实现A∪B的集合运算:
1. 定义三个数组a、b、c,分别表示集合A、B、C。
2. 初始化数组a、b、c为0。
3. 读入集合A中的元素,将a数组中对应位置赋值为1。
4. 读入集合B中的元素,将b数组中对应位置赋值为1。
5. 对于数组c中的每个元素i,将c[i]赋值为a[i]或b[i],即c[i] = a[i] || b[i]。
6. 遍历数组c,统计其中的1的个数,即为集合C的元素个数。
以下是示例代码:
```c
#include <stdio.h>
#define MAX_SIZE 100
void set_union(int a[], int b[], int c[]) {
int i;
for (i = 0; i < MAX_SIZE; i++) {
c[i] = a[i] || b[i];
}
}
int count_set(int c[]) {
int count = 0, i;
for (i = 0; i < MAX_SIZE; i++) {
if (c[i]) {
count++;
}
}
return count;
}
int main() {
int a[MAX_SIZE] = {0}, b[MAX_SIZE] = {0}, c[MAX_SIZE] = {0};
int n, m, x, i;
printf("Enter the number of elements in set A: ");
scanf("%d", &n);
printf("Enter the elements of set A: ");
for (i = 0; i < n; i++) {
scanf("%d", &x);
a[x] = 1;
}
printf("Enter the number of elements in set B: ");
scanf("%d", &m);
printf("Enter the elements of set B: ");
for (i = 0; i < m; i++) {
scanf("%d", &x);
b[x] = 1;
}
set_union(a, b, c);
printf("The elements in set C (A union B) are: ");
for (i = 0; i < MAX_SIZE; i++) {
if (c[i]) {
printf("%d ", i);
}
}
printf("\n");
printf("The number of elements in set C is: %d\n", count_set(c));
return 0;
}
```
注意:该代码假设集合中的元素均为非负整数。如果集合中包含负整数,需要对数组下标进行偏移。
阅读全文
相关推荐













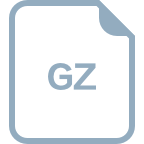
