priority_queue简单使用例子
时间: 2023-07-08 19:38:55 浏览: 109
下面是一个简单的使用 C++ STL 中的 priority_queue 的例子:
```c++
#include <iostream>
#include <queue>
using namespace std;
int main() {
priority_queue<int> pq;
pq.push(10);
pq.push(30);
pq.push(20);
while (!pq.empty()) {
cout << pq.top() << " ";
pq.pop();
}
return 0;
}
```
输出结果为:
```
30 20 10
```
在这个例子中,我们创建了一个 `priority_queue` 对象 `pq`,并使用 `push()` 方法添加了三个整数到队列中。然后,我们使用 `while` 循环遍历队列中的元素,每次输出队列中的最大值(默认情况下,`priority_queue` 是一个最大堆),并使用 `pop()` 方法弹出最大值,直到队列为空。
相关问题
举几个使用 priority_queue的代码例子
以下是三个使用 priority_queue 的代码例子:
1. 求一组数中的前 k 大元素
```c++
int main() {
int n = 10, k = 3;
vector<int> nums = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3};
priority_queue<int, vector<int>, greater<int>> pq;
for (int i = 0; i < n; i++) {
pq.push(nums[i]);
if (pq.size() > k) pq.pop();
}
while (!pq.empty()) {
cout << pq.top() << " ";
pq.pop();
}
return 0;
}
```
2. 最小的 k 个数
```c++
int main() {
int n = 10, k = 3;
vector<int> nums = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3};
priority_queue<int> pq;
for (int i = 0; i < n; i++) {
pq.push(nums[i]);
if (pq.size() > k) pq.pop();
}
while (!pq.empty()) {
cout << pq.top() << " ";
pq.pop();
}
return 0;
}
```
3. 利用优先队列实现堆排序
```c++
void heap_sort(vector<int>& nums) {
int n = nums.size();
priority_queue<int, vector<int>, greater<int>> pq;
for (int i = 0; i < n; i++) {
pq.push(nums[i]);
}
int i = 0;
while (!pq.empty()) {
nums[i++] = pq.top();
pq.pop();
}
}
```
c++ std::priority_queue使用例子
以下是一个简单的使用std::priority_queue的例子:
```c++
#include <iostream>
#include <queue> // 包含 priority_queue 头文件
using namespace std;
int main() {
// 定义一个最大堆
priority_queue<int> max_heap;
// 插入元素
max_heap.push(10);
max_heap.push(30);
max_heap.push(20);
// 访问堆顶元素
cout << "堆顶元素: " << max_heap.top() << endl;
// 删除堆顶元素
max_heap.pop();
// 访问堆顶元素
cout << "删除堆顶元素后,新的堆顶元素: " << max_heap.top() << endl;
// 获取堆中元素个数
cout << "堆中元素个数: " << max_heap.size() << endl;
// 判断堆是否为空
if (max_heap.empty()) {
cout << "堆为空" << endl;
} else {
cout << "堆不为空" << endl;
}
return 0;
}
```
输出结果:
```
堆顶元素: 30
删除堆顶元素后,新的堆顶元素: 20
堆中元素个数: 2
堆不为空
```
在这个例子中,我们定义了一个最大堆,并向其中插入了三个元素。我们使用top()函数来访问堆顶元素,并使用pop()函数删除堆顶元素。最后,我们使用size()函数来获取堆中元素的个数,使用empty()函数来判断堆是否为空。
阅读全文
相关推荐
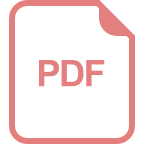













