利用python构建一个BP神经网络(附件为BP.py代码)并训练异或数据样本,并以图像形式呈现
时间: 2024-05-16 14:12:22 浏览: 177
好的,我会帮您解决这个问题。
首先,您需要准备以下数据:
```python
import numpy as np
# 定义异或数据样本
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
y = np.array([[0], [1], [1], [0]])
```
然后,您需要构建一个BP神经网络。请参考如下代码:
```python
class NeuralNetwork:
def __init__(self, layers):
self.layers = layers
self.weights = []
self.biases = []
for i in range(len(layers) - 1):
w = np.random.randn(layers[i], layers[i+1])
b = np.random.randn(layers[i+1])
self.weights.append(w)
self.biases.append(b)
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(self, x):
return x * (1 - x)
def feedforward(self, x):
a = x
for w, b in zip(self.weights, self.biases):
z = np.dot(a, w) + b
a = self.sigmoid(z)
return a
def backpropagation(self, x, y, learning_rate):
# 前向传递
a = x
activations = [a]
zs = []
for w, b in zip(self.weights, self.biases):
z = np.dot(a, w) + b
zs.append(z)
a = self.sigmoid(z)
activations.append(a)
# 反向传递
delta = (activations[-1] - y) * self.sigmoid_derivative(activations[-1])
deltas = [delta]
for i in range(len(self.layers)-2):
delta = np.dot(deltas[-1], self.weights[-i-1].T) * self.sigmoid_derivative(activations[-2-i])
deltas.append(delta)
deltas.reverse()
# 更新权重和偏差
for i in range(len(self.weights)):
w_grad = np.dot(activations[i].T, deltas[i])
b_grad = np.sum(deltas[i], axis=0)
self.weights[i] -= learning_rate * w_grad
self.biases[i] -= learning_rate * b_grad
def train(self, X, y, epochs, learning_rate):
for i in range(epochs):
for j in range(len(X)):
self.backpropagation(X[j], y[j], learning_rate)
```
接下来,您需要实例化神经网络并进行训练。请参考如下代码:
```python
# 实例化神经网络
nn = NeuralNetwork([2, 4, 1])
# 训练神经网络
nn.train(X, y, epochs=10000, learning_rate=0.1)
```
最后,您需要将训练后的结果可视化。请参考如下代码:
```python
import matplotlib.pyplot as plt
# 可视化训练结果
x_min, x_max = -0.5, 1.5
y_min, y_max = -0.5, 1.5
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.01),
np.arange(y_min, y_max, 0.01))
Z = np.array([nn.feedforward(np.array([[x, y]]))[0][0] for x, y in np.c_[xx.ravel(), yy.ravel()]])
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, cmap=plt.cm.Spectral)
plt.scatter(X[:,0], X[:,1], c=y.ravel(), cmap=plt.cm.Spectral)
plt.show()
```
这样,您就可以看到训练结果了。完整代码如下:
阅读全文
相关推荐
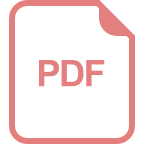
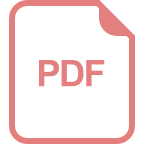
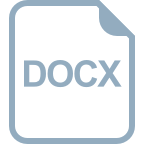
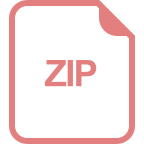
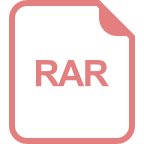
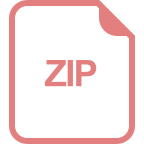
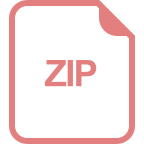
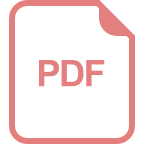
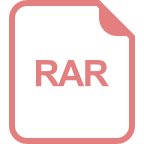
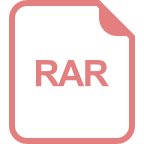
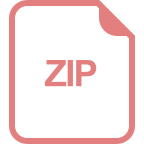
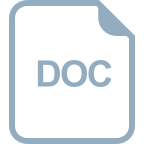
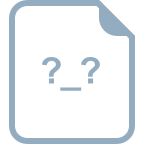
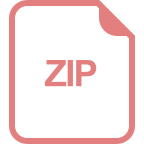
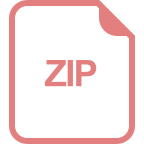