python装饰器,常用装饰器及其使用方法
时间: 2023-07-11 13:51:32 浏览: 105
常用的Python装饰器有以下几种:
1. @staticmethod:静态方法装饰器,用于声明一个静态方法。
```python
class MyClass:
@staticmethod
def my_static_method():
print("This is a static method.")
```
2. @classmethod:类方法装饰器,用于声明一个类方法。
```python
class MyClass:
@classmethod
def my_class_method(cls):
print("This is a class method.")
```
3. @property:属性装饰器,用于将方法转换为只读属性。
```python
class MyClass:
def __init__(self, value):
self._value = value
@property
def value(self):
return self._value
```
4. @abstractmethod:抽象方法装饰器,用于声明一个抽象方法。
```python
from abc import ABC, abstractmethod
class MyAbstractClass(ABC):
@abstractmethod
def my_abstract_method(self):
pass
```
5. @wraps:装饰器装饰器,用于保留被装饰函数的元信息。
```python
from functools import wraps
def my_decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
print("Before the function is called.")
result = func(*args, **kwargs)
print("After the function is called.")
return result
return wrapper
@my_decorator
def say_hello():
"""A function that says hello."""
print("Hello!")
```
以上是常用的Python装饰器及其使用方法。
阅读全文
相关推荐
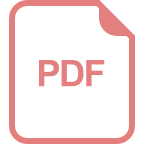
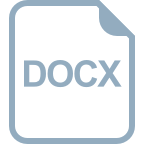
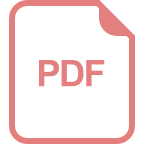
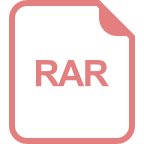
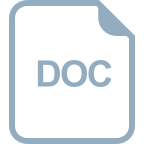
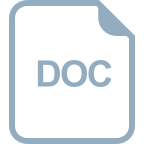
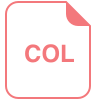
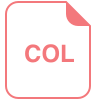
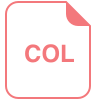
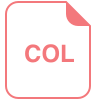
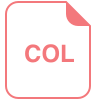
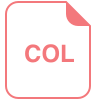
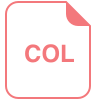
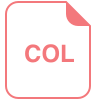
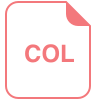
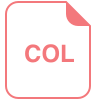
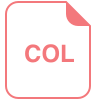