Python OOP设计模式:理解常用模式及其应用,构建可扩展且灵活的代码
发布时间: 2024-06-25 16:54:39 阅读量: 70 订阅数: 35 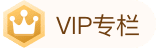
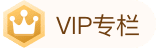
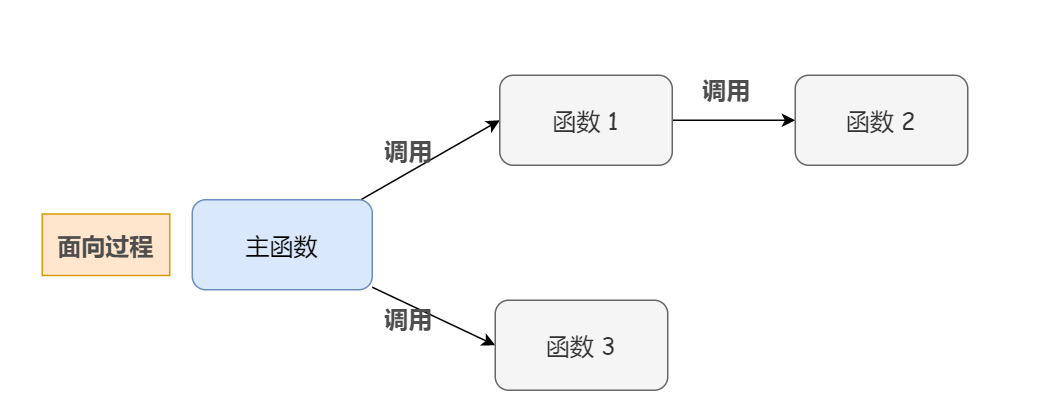
# 1. 面向对象编程(OOP)的基础**
面向对象编程(OOP)是一种编程范式,它以对象为中心,对象包含数据(属性)和操作数据的方法(方法)。OOP 提供了封装、继承和多态性等特性,这些特性使软件开发更具可扩展性、可维护性和可重用性。
OOP 的基本概念包括:
- **类:** 类的模板,定义对象的属性和方法。
- **对象:** 类的实例,具有自己的属性和方法。
- **封装:** 隐藏对象的内部细节,只公开必要的接口。
- **继承:** 子类继承父类的属性和方法,实现代码重用。
- **多态性:** 子类可以重写父类的方法,实现不同的行为。
# 2.1 创建型模式
### 2.1.1 工厂模式
工厂模式是一种创建型设计模式,它提供了一个创建对象的接口,而不指定创建对象的具体类。这种模式允许系统在不修改客户端代码的情况下更改创建对象的类。
**代码块:**
```python
class ShapeFactory:
def get_shape(self, shape_type):
if shape_type == "Circle":
return Circle()
elif shape_type == "Square":
return Square()
elif shape_type == "Rectangle":
return Rectangle()
else:
raise ValueError("Invalid shape type")
class Circle:
def draw(self):
print("Drawing a circle")
class Square:
def draw(self):
print("Drawing a square")
class Rectangle:
def draw(self):
print("Drawing a rectangle")
# 使用工厂模式
factory = ShapeFactory()
circle = factory.get_shape("Circle")
circle.draw()
```
**逻辑分析:**
* `ShapeFactory` 类提供了一个 `get_shape` 方法,它根据给定的形状类型返回一个形状对象。
* 客户端代码通过 `ShapeFactory` 类创建形状对象,而无需知道具体创建哪种形状类。
* 工厂模式允许系统在不修改客户端代码的情况下更改创建对象的类。
### 2.1.2 单例模式
单例模式是一种创建型设计模式,它确保一个类只有一个实例。这种模式用于创建全局对象,例如数据库连接或缓存。
**代码块:**
```python
class Singleton:
_instance = None
def __new__(cls, *args, **kwargs):
if not cls._instance:
cls._instance = super(Singleton, cls).__new__(cls, *args, **kwargs)
return cls._instance
# 使用单例模式
singleton1 = Singleton()
singleton2 = Singleton()
print(singleton1 is singleton2) # True
```
**逻辑分析:**
* `Singleton` 类使用 `__new__` 方法来控制实例的创建。
* 如果类还没有实例,则 `__new__` 方法创建一个新的实例并将其存储在 `_instance` 属性中。
* 如果类已经有一个实例,则 `__new__` 方法返回现有实例。
* 客户端代码通过调用 `Singleton` 类来
0
0
相关推荐
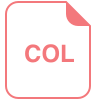
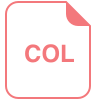
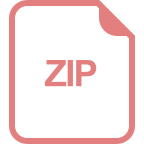
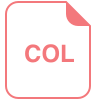
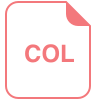
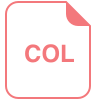