神经网络的Python实现代码
时间: 2023-11-19 11:14:18 浏览: 34
好的,以下是一个简单的神经网络的Python实现代码,它可以实现对手写数字进行识别:
```python
import numpy as np
# 定义sigmoid函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义神经网络的类
class NeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
# 随机初始化权重
self.weights1 = np.random.randn(self.hidden_size, self.input_size)
self.weights2 = np.random.randn(self.output_size, self.hidden_size)
def forward(self, x):
# 计算第一层的输出
self.layer1 = sigmoid(np.dot(self.weights1, x))
# 计算输出层的输出
self.layer2 = sigmoid(np.dot(self.weights2, self.layer1))
return self.layer2
def backward(self, x, y, output):
# 计算输出层的误差
error = output - y
# 计算输出层的梯度
output_gradient = error * output * (1 - output)
# 计算第一层的误差和梯度
layer1_error = np.dot(self.weights2.T, output_gradient)
layer1_gradient = self.layer1 * (1 - self.layer1) * layer1_error
# 更新权重
self.weights2 -= 0.1 * np.outer(output_gradient, self.layer1)
self.weights1 -= 0.1 * np.outer(layer1_gradient, x)
def train(self, x, y):
output = self.forward(x)
self.backward(x, y, output)
# 加载手写数字数据集
from sklearn.datasets import load_digits
digits = load_digits()
# 神经网络的输入和输出
x = digits.images.reshape((len(digits.images), -1)).T
y = digits.target
# 对输出进行one-hot编码
y_onehot = np.zeros((len(y), 10))
for i in range(len(y)):
y_onehot[i, y[i]] = 1
# 创建神经网络
nn = NeuralNetwork(64, 16, 10)
# 训练神经网络
for i in range(1000):
for j in range(len(x)):
nn.train(x[:, j], y_onehot[j])
# 测试神经网络
correct = 0
for i in range(len(x)):
output = nn.forward(x[:, i])
if np.argmax(output) == y[i]:
correct += 1
# 输出识别准确率
print("Accuracy:", correct / len(x))
```
这段代码使用了numpy库来进行矩阵运算,实现了一个包含一个隐层的全连接神经网络,用于识别手写数字。
相关推荐
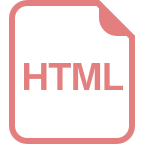
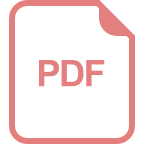














