在vue2中使用three.js生成一个大型模型
时间: 2024-05-15 10:19:51 浏览: 9
在Vue2中使用Three.js生成一个大型模型需要以下步骤:
1. 安装Three.js
可以通过npm安装Three.js
```
npm install three
```
2. 在Vue组件中引入Three.js
在Vue组件中引入Three.js
```javascript
import * as THREE from 'three'
```
3. 创建一个Three.js场景
```javascript
mounted() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 创建渲染器
this.renderer = new THREE.WebGLRenderer();
this.renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(this.renderer.domElement);
}
```
4. 创建一个大型模型
```javascript
mounted() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 创建渲染器
this.renderer = new THREE.WebGLRenderer();
this.renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(this.renderer.domElement);
// 创建几何体
const geometry = new THREE.BoxGeometry(1, 1, 1);
// 创建材质
const material = new THREE.MeshBasicMaterial({
color: 0x00ff00
});
// 创建网格
this.cube = new THREE.Mesh(geometry, material);
// 将网格添加到场景中
this.scene.add(this.cube);
// 设置相机位置
this.camera.position.z = 5;
}
```
5. 渲染场景
```javascript
mounted() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 创建渲染器
this.renderer = new THREE.WebGLRenderer();
this.renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(this.renderer.domElement);
// 创建几何体
const geometry = new THREE.BoxGeometry(1, 1, 1);
// 创建材质
const material = new THREE.MeshBasicMaterial({
color: 0x00ff00
});
// 创建网格
this.cube = new THREE.Mesh(geometry, material);
// 将网格添加到场景中
this.scene.add(this.cube);
// 设置相机位置
this.camera.position.z = 5;
// 渲染场景
const animate = () => {
requestAnimationFrame(animate);
this.cube.rotation.x += 0.01;
this.cube.rotation.y += 0.01;
this.renderer.render(this.scene, this.camera);
};
animate();
}
```
以上就是在Vue2中使用Three.js生成一个大型模型的步骤。具体的实现可以根据需求进行调整。
相关推荐
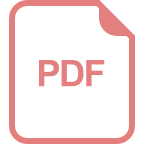











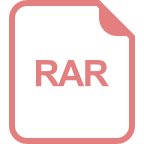
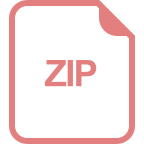
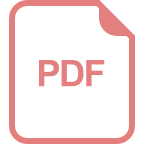