c语言实现在tcp协议下实现实时的编码视频传输代码
时间: 2024-02-29 07:55:59 浏览: 25
实时的编码视频传输代码需要使用视频编码库(例如FFmpeg)和网络编程库(例如socket)。以下是一个基本的C语言示例代码,可以实现在TCP协议下实时编码视频传输:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#define PORT 8888
#define WIDTH 640
#define HEIGHT 480
int main(int argc, char *argv[]) {
int sockfd, newsockfd, clilen;
struct sockaddr_in serv_addr, cli_addr;
int width = WIDTH, height = HEIGHT, fps = 25;
AVCodecContext *codecCtx = NULL;
AVFormatContext *formatCtx = NULL;
AVInputFormat *inputFormat = NULL;
AVOutputFormat *outputFormat = NULL;
AVStream *inStream = NULL, *outStream = NULL;
AVCodec *codec = NULL;
AVFrame *frame = NULL, *frameRGB = NULL;
AVPacket pkt;
int frameCount = 0;
struct SwsContext *swsCtx = NULL;
uint8_t *buffer = NULL;
int numBytes;
int ret;
// 初始化网络
sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("ERROR opening socket");
exit(1);
}
bzero((char *) &serv_addr, sizeof(serv_addr));
serv_addr.sin_family = AF_INET;
serv_addr.sin_addr.s_addr = INADDR_ANY;
serv_addr.sin_port = htons(PORT);
if (bind(sockfd, (struct sockaddr *) &serv_addr, sizeof(serv_addr)) < 0) {
perror("ERROR on binding");
exit(1);
}
listen(sockfd, 5);
clilen = sizeof(cli_addr);
newsockfd = accept(sockfd, (struct sockaddr *) &cli_addr, &clilen);
if (newsockfd < 0) {
perror("ERROR on accept");
exit(1);
}
// 初始化视频编码器
av_register_all();
avcodec_register_all();
codec = avcodec_find_encoder(AV_CODEC_ID_H264);
codecCtx = avcodec_alloc_context3(codec);
codecCtx->bit_rate = 400000;
codecCtx->width = width;
codecCtx->height = height;
codecCtx->time_base.num = 1;
codecCtx->time_base.den = fps;
codecCtx->gop_size = 10;
codecCtx->max_b_frames = 1;
codecCtx->pix_fmt = AV_PIX_FMT_YUV420P;
if (avcodec_open2(codecCtx, codec, NULL) < 0) {
perror("ERROR opening codec");
exit(1);
}
// 初始化视频输入和输出
avformat_alloc_output_context2(&formatCtx, NULL, "mpegts", NULL);
outputFormat = formatCtx->oformat;
inputFormat = av_find_input_format("video4linux2");
if (avformat_open_input(&formatCtx, "/dev/video0", inputFormat, NULL) != 0) {
perror("ERROR opening input");
exit(1);
}
if (avformat_find_stream_info(formatCtx, NULL) < 0) {
perror("ERROR finding stream info");
exit(1);
}
av_dump_format(formatCtx, 0, "/dev/video0", 0);
inStream = formatCtx->streams[0];
outStream = avformat_new_stream(formatCtx, codec);
outStream->id = 0;
if (avcodec_copy_context(outStream->codec, codecCtx) < 0) {
perror("ERROR copying codec context");
exit(1);
}
outStream->codec->codec_tag = 0;
if (formatCtx->oformat->flags & AVFMT_GLOBALHEADER) {
outStream->codec->flags |= CODEC_FLAG_GLOBAL_HEADER;
}
// 初始化RGB帧和转换器
frame = av_frame_alloc();
frameRGB = av_frame_alloc();
numBytes = avpicture_get_size(AV_PIX_FMT_RGB24, width, height);
buffer = (uint8_t *) av_malloc(numBytes * sizeof(uint8_t));
avpicture_fill((AVPicture *) frameRGB, buffer, AV_PIX_FMT_RGB24, width, height);
swsCtx = sws_getContext(width, height, inStream->codec->pix_fmt,
width, height, AV_PIX_FMT_RGB24, SWS_BILINEAR,
NULL, NULL, NULL);
// 开始传输
avformat_write_header(formatCtx, NULL);
while (1) {
if (av_read_frame(formatCtx, &pkt) >= 0) {
if (pkt.stream_index == 0) {
avcodec_decode_video2(inStream->codec, frame, &ret, &pkt);
if (ret) {
sws_scale(swsCtx, frame->data, frame->linesize, 0, height, frameRGB->data, frameRGB->linesize);
av_init_packet(&pkt);
pkt.data = NULL;
pkt.size = 0;
avcodec_encode_video2(outStream->codec, &pkt, frameRGB, &ret);
if (ret) {
pkt.stream_index = outStream->index;
av_write_frame(formatCtx, &pkt);
}
}
}
av_free_packet(&pkt);
}
if (++frameCount == fps * 30) {
break;
}
}
av_write_trailer(formatCtx);
// 清理
close(newsockfd);
close(sockfd);
av_free(buffer);
av_free(frameRGB);
av_free(frame);
avcodec_close(codecCtx);
avformat_close_input(&formatCtx);
return 0;
}
```
这个例子使用Video4Linux2作为输入源,将视频编码为H.264格式,并通过TCP协议流式传输。你可以根据自己的需求修改代码。
相关推荐
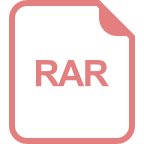
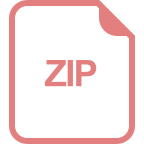
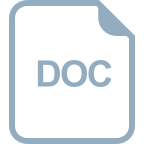














