RANSAC拟合光平面python实现实列
时间: 2023-10-03 17:07:27 浏览: 47
RANSAC是一种鲁棒性较强的拟合算法,可以用于拟合平面等几何形状。这里给出一个用Python实现RANSAC拟合平面的示例代码。
```
import numpy as np
import random
def fit_plane_RANSAC(points, max_iterations=1000, distance_threshold=0.01):
"""
RANSAC algorithm for fitting a plane to a set of 3D points.
:param points: A Nx3 numpy array of 3D points.
:param max_iterations: The maximum number of iterations to run RANSAC.
:param distance_threshold: The maximum distance from a point to the plane for it to be considered an inlier.
:return: A tuple of (best_plane, best_inliers) where best_plane is a length-4 numpy array representing the plane
equation in the form [a, b, c, d] (ax + by + cz + d = 0), and best_inliers is a boolean numpy array of length N
indicating which points were classified as inliers.
"""
best_plane = None
best_inliers = None
best_num_inliers = 0
for i in range(max_iterations):
# Randomly sample 3 points from the set of points
sample_indices = random.sample(range(points.shape[0]), 3)
p1, p2, p3 = points[sample_indices]
# Compute the plane equation from the 3 points
v1 = p2 - p1
v2 = p3 - p1
normal = np.cross(v1, v2)
d = -np.dot(normal, p1)
plane = np.array([normal[0], normal[1], normal[2], d])
# Compute the distance from each point to the plane
distances = np.abs(np.dot(points, plane[:3]) + plane[3]) / np.linalg.norm(plane[:3])
# Count the number of inliers (points within the distance threshold)
inliers = distances < distance_threshold
num_inliers = np.count_nonzero(inliers)
# Check if this is the best model so far
if num_inliers > best_num_inliers:
best_plane = plane
best_inliers = inliers
best_num_inliers = num_inliers
return best_plane, best_inliers
```
这个函数的输入是一个 Nx3 的numpy数组,代表N个3D点。max_iterations和distance_threshold分别是RANSAC算法的最大迭代次数和点到平面的最大距离阈值。函数的输出是一个二元组,第一个元素是拟合出来的平面方程(以[a, b, c, d]的形式表示),第二个元素是一个长度为N的布尔型numpy数组,表示每个点是否被分类为内点(True)或外点(False)。
相关推荐
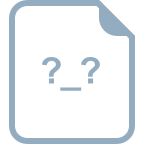













