python基于RSA算法的非对称数字签名,要求,p,q不需要大素数,普通素数即可,但p,q,e要求为可输入数字,认证信息为任意可输入数字
时间: 2023-10-14 11:06:01 浏览: 86
RSA算法的非对称数字签名包括私钥生成、签名和验证三个步骤。下面是Python实现代码:
私钥生成:
```python
import random
def gcd(a, b):
while b != 0:
a, b = b, a % b
return a
def mod_inverse(e, phi):
x1, y1, x2, y2 = 1, 0, 0, 1
while phi != 0:
q, e, phi = e // phi, phi, e % phi
x1, x2 = x2, x1 - q * x2
y1, y2 = y2, y1 - q * y2
return e, x1, y1
def generate_key(p, q, e):
n = p * q
phi = (p - 1) * (q - 1)
gcd_value = gcd(e, phi)
if gcd_value != 1:
raise ValueError("e is not coprime with phi")
d = mod_inverse(e, phi)[1]
return ((n, e), (n, d))
```
签名:
```python
def sign(private_key, message):
n, d = private_key
return pow(message, d, n)
```
验证:
```python
def verify(public_key, message, signature):
n, e = public_key
return message == pow(signature, e, n)
```
示例:
```python
# 生成公私钥
p = 11
q = 17
e = 7
public_key, private_key = generate_key(p, q, e)
# 生成认证信息
message = 123456789
# 签名
signature = sign(private_key, message)
# 验证
assert verify(public_key, message, signature)
```
需要注意的是,这里的p、q、e都是可输入的普通数字,因此没有进行素性检测,生成的密钥可能不是安全的。在实际使用中,应该使用大素数,并进行素性检测。
阅读全文
相关推荐
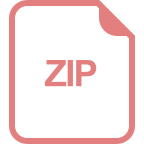
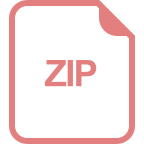



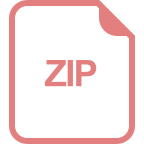
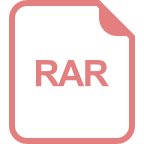
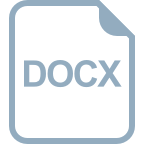
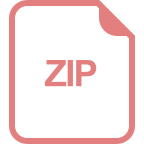
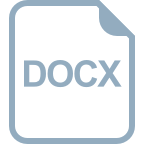

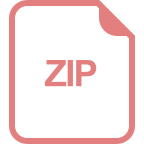
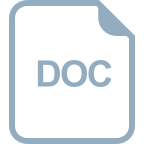


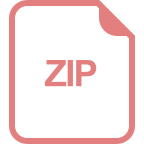