写出matlab中距离变化使用函数代码
时间: 2023-05-22 10:07:07 浏览: 97
Sure, here's an example code for the distance function in MATLAB:
```matlab
% Create two arrays representing two points in 3D space
point1 = [3, 5, 2];
point2 = [-1, 2, 6];
% Calculate the Euclidean distance between the points
distance = norm(point1 - point2);
```
The `norm` function calculates the Euclidean distance between two points, which is simply the length of the line connecting the points in 3D space. This code should work for any two points in 3D space.
相关问题
请根据你说的步骤写出MATLAB代码
根据你提供的SAR成像参数,以下是一个简单的MATLAB代码示例,用于实现SAR图像重建:
```matlab
% 读取原始回波数据
data = fread(fid, [2, N], 'uint8'); % 假设原始数据为[N,2]的矩阵,其中第一列为实部,第二列为虚部
data = data(1,:) + 1j*data(2,:); % 将实部和虚部合成复数值
% 距离向压缩
win = kaiser(N, beta); % 数据窗函数
data = data .* win'; % 加窗
data = fft(data); % FFT变换
% 多普勒向压缩
fd = linspace(-0.5, 0.5, N) * prf; % 多普勒频率
f0 = -doppler_center; % 多普勒中心频率
data = data .* exp(-1j*2*pi*fd*(f0/prf)); % 相移
data = fftshift(data); % 频域移位
data = sum(reshape(data, [], num_lines), 2); % 多普勒向累加
% 逆FFT变换
data = ifft(data); % 逆FFT变换
% SAR图像重建
range = linspace(0, c/2/B, N); % 距离向采样点
azimuth = linspace(-pi/2, pi/2, num_lines); % 方位向采样点
[X, Y] = meshgrid(azimuth, range); % 采样网格
data = reshape(data, num_lines, []); % 转换为[num_lines, N/num_lines]的矩阵
figure, imagesc(azimuth, range, abs(data)); % 显示SAR图像
```
注:以上代码仅为演示用途,实际应用中可能需要更多的错误处理和优化。另外,其中的变量和参数需要根据具体情况进行调整。
mean shift 进行水果图像分割 使用matlab 写出具体代码
好的,针对您的问题,我先解释一下mean shift的原理。mean shift是一种图像分割算法,主要是利用像素点的颜色和空间信息进行聚类,通常用于图像的分割和目标跟踪。在mean shift算法中,首先需要定义像素点的颜色和空间距离,然后计算每个像素点的核密度估计。接下来,对于每个像素点,计算出它在核密度函数下的聚类中心,不断地对每个点的聚类中心进行迭代,最终得到每个点所在的聚类中心,从而实现图像的分割。
那么关于您提到的具体matlab代码实现,可以参考以下步骤:
1. 读取图像并转换成Lab色彩空间
```
img = imread('fruit.jpg');
[rows, cols, channels] = size(img);
img_Lab = rgb2lab(img);
```
2. 初始化 mean shift 算法所需的参数,包括颜色和空间的窗口大小、核密度函数的带宽等
```
window_size = 30;
sigma = 10;
L = img_Lab(:,:,1);
a = img_Lab(:,:,2);
b = img_Lab(:,:,3);
[X,Y] = meshgrid(1:cols,1:rows);
XY = [X(:),Y(:)];
```
3. 对于每个像素点,计算出它的核密度函数和聚类中心坐标,并将所属聚类中心的像素点分类
```
for i = 1:numel(XY)/2
data_space = [X(:)-XY(i,1), Y(:)-XY(i,2)];
distance_space = sqrt(data_space(:,1).^2 + data_space(:,2).^2);
data_color = [L(:), a(:), b(:)];
distance_color = sqrt(sum((data_color - repmat(data_color(i,:),rows*cols,1)).^2,2));
distance_total = sqrt(distance_color.^2 + (distance_space/window_size).^2);
kernel = exp(-(distance_total.^2)./sigma^2);
[max_kernel, c_idx] = max(kernel);
center = [L(c_idx) a(c_idx) b(c_idx)];
centers(i,:) = center;
end
[~, pixels_cluster_idx] = max(kernel');
```
4. 对分割后的图像进行可视化
```
for j=1:size(centers,1);
mask = pixels_cluster_idx(:) == j;
img_out = img;
img_out(repmat(~mask,[1 1 3])) = 0;
figure,imshow(img_out);
title(['Image after mean-shift segmentation, cluster:',num2str(j)])
end
```
以上就是一个简单的mean shift图像分割的代码实现,希望能有所帮助。
相关推荐
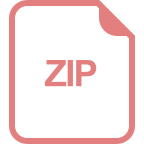












