编写一个代码stm32f103通过dht11采集温度当温度大于三十的时候蜂鸣器响分为主函数和.c和.h文件
时间: 2024-02-20 10:01:08 浏览: 75
以下是主函数的代码:
```c
#include "stm32f10x.h"
#include "dht11.h"
#include "delay.h"
#include "beep.h"
int main()
{
uint8_t temperature;
BEEP_Init();
DHT11_Init();
while(1)
{
if(DHT11_Read_Temperature(&temperature) == SUCCESS)
{
if(temperature > 30)
{
BEEP_On();
}
else
{
BEEP_Off();
}
}
Delay_Ms(1000);
}
}
```
这里使用了三个外部库,分别是dht11.h和dht11.c(用于驱动DHT11传感器)、delay.h和delay.c(用于延时函数)和beep.h和beep.c(用于驱动蜂鸣器)。以下是dht11.h和dht11.c的代码:
dht11.h:
```c
#ifndef __DHT11_H
#define __DHT11_H
#include "stm32f10x.h"
typedef enum
{
SUCCESS = 0,
ERROR = 1
} Status_TypeDef;
void DHT11_Init(void);
Status_TypeDef DHT11_Read_Temperature(uint8_t *temperature);
#endif /* __DHT11_H */
```
dht11.c:
```c
#include "dht11.h"
#define DHT11_PIN GPIO_Pin_0
#define DHT11_PORT GPIOA
#define DHT11_RCC RCC_APB2Periph_GPIOA
void DHT11_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(DHT11_RCC, ENABLE);
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
}
static uint8_t DHT11_Read_Byte(void)
{
uint8_t i, j;
uint8_t byte = 0;
for(i = 0; i < 8; i++)
{
while(!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN));
Delay_Us(30);
if(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN))
{
byte |= (1 << (7 - i));
}
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN));
}
return byte;
}
Status_TypeDef DHT11_Read_Temperature(uint8_t *temperature)
{
uint8_t data[5];
uint8_t i;
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DHT11_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
GPIO_ResetBits(DHT11_PORT, DHT11_PIN);
Delay_Ms(18);
GPIO_SetBits(DHT11_PORT, DHT11_PIN);
Delay_Us(40);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(DHT11_PORT, &GPIO_InitStructure);
if(!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN))
{
while(!GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN));
while(GPIO_ReadInputDataBit(DHT11_PORT, DHT11_PIN));
for(i = 0; i < 5; i++)
{
data[i] = DHT11_Read_Byte();
}
if((data[0] + data[1] + data[2] + data[3]) == data[4])
{
*temperature = data[2];
return SUCCESS;
}
}
return ERROR;
}
```
以下是delay.h和delay.c的代码:
delay.h:
```c
#ifndef __DELAY_H
#define __DELAY_H
#include "stm32f10x.h"
void Delay_Init(void);
void Delay_Us(uint32_t us);
void Delay_Ms(uint32_t ms);
#endif /* __DELAY_H */
```
delay.c:
```c
#include "delay.h"
static uint32_t usTicks;
void Delay_Init(void)
{
SysTick_Config(SystemCoreClock / 1000000);
}
void SysTick_Handler(void)
{
if(usTicks != 0)
{
usTicks--;
}
}
void Delay_Us(uint32_t us)
{
usTicks = us;
while(usTicks);
}
void Delay_Ms(uint32_t ms)
{
while(ms--)
{
Delay_Us(1000);
}
}
```
最后,以下是beep.h和beep.c的代码:
beep.h:
```c
#ifndef __BEEP_H
#define __BEEP_H
#include "stm32f10x.h"
void BEEP_Init(void);
void BEEP_On(void);
void BEEP_Off(void);
#endif /* __BEEP_H */
```
beep.c:
```c
#include "beep.h"
#define BEEP_PIN GPIO_Pin_1
#define BEEP_PORT GPIOA
#define BEEP_RCC RCC_APB2Periph_GPIOA
void BEEP_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(BEEP_RCC, ENABLE);
GPIO_InitStructure.GPIO_Pin = BEEP_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(BEEP_PORT, &GPIO_InitStructure);
}
void BEEP_On(void)
{
GPIO_SetBits(BEEP_PORT, BEEP_PIN);
}
void BEEP_Off(void)
{
GPIO_ResetBits(BEEP_PORT, BEEP_PIN);
}
```
阅读全文
相关推荐
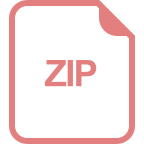
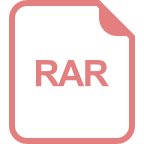
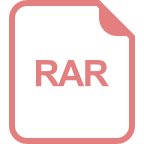
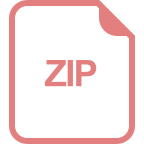
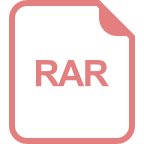
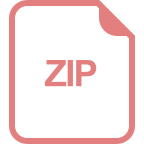
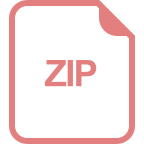
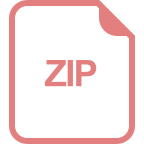
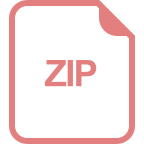
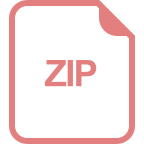






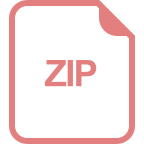
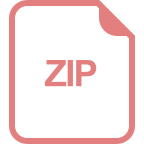