#include <iostream> using namespace std; class MyInt { private: int n; public: MyInt() {} MyInt(int n) :n(n) {} MyInt& operator ++()//前置++ { n++; return *this; } const MyInt operator ++(int)//后置++ { MyInt temp(*this); n++; return temp; } void display() { cout << n << endl; } }; int main() { MyInt t1(5), t2, t3; t2 = ++t1; t1.display(); t2.display(); t3 = t2++; t2.display(); t3.display(); return 0; }逐句解析
时间: 2024-04-07 11:28:16 浏览: 24
这段代码是一个简单的 C++ 程序,主要演示了前置和后置自增运算符的重载。
首先,包含了头文件 iostream,用于输入输出。使用了命名空间 std。
接下来,定义了一个名为 MyInt 的类,其中包含了一个私有成员变量 n 和三个公有成员函数:一个默认构造函数、一个带参数的构造函数、以及一个重载了前置和后置自增运算符的函数。
重载前置自增运算符的函数返回了一个 MyInt 类型的引用,将 n 的值加一,并返回自身对象的引用。
重载后置自增运算符的函数返回了一个 const MyInt 类型的对象,首先将自身对象拷贝一份到一个临时对象 temp 中,然后将 n 的值加一,并返回这个临时对象 temp。
最后,定义了主函数 main,其中定义了三个 MyInt 类型的对象 t1、t2 和 t3。首先用 t1 初始化 t2,将 t1 的值加一并赋值给 t2。然后用 t2 初始化 t3,将 t2 的值加一并赋值给 t3。最后输出 t1、t2 和 t3 的值。
运行结果为:
6
6
7
6
解释:
首先,t1 的初始值为 5,调用前置自增运算符后 t1 的值变为 6,然后将 t1 赋值给 t2,所以 t2 的值也为 6,输出 t1 和 t2 的值均为 6。
然后,将 t2 赋值给 t3,调用后置自增运算符后 t2 的值变为 7,但 t3 的值还是之前的 6,输出 t2 和 t3 的值分别为 7 和 6。
相关问题
【填空题】下面吥MyInt类只吽吺个数据成员,请将呉序补充完整。 #include <iostream> using namespace std; class MyInt { int nVal; public: MyInt(int n) : nVal(n) { } int ReturnVal() { return nVal; } // 吖吥妠码在吷里 }; int main() { MyInt objInt(10); objInt - 2 - 1 - 3; cout << objInt.ReturnVal(); cout << ","; objInt - 2 - 1; cout << objInt.ReturnVal(); return 0; } 输出: 4,1
下面是MyInt类完整的实现代码:
```c++
#include <iostream>
using namespace std;
class MyInt {
int nVal;
public:
MyInt(int n) : nVal(n) { }
int ReturnVal() { return nVal; }
MyInt& operator-(int n) {
nVal -= n;
return *this;
}
};
int main() {
MyInt objInt(10);
objInt - 2 - 1 - 3;
cout << objInt.ReturnVal();
cout << ",";
objInt - 2 - 1;
cout << objInt.ReturnVal();
return 0;
}
```
解析:
- MyInt类中添加了一行代码,重载了减号运算符(operator-)。
- 减号运算符返回的是MyInt类对象的引用,因此可以连续进行多次减法运算。
- 在main函数中,依次对objInt进行了三次减法运算,最终输出结果为4和1。
C++中的tuple
C++中的tuple是一个固定大小的不同类型元素的集合,类似于结构体,但是tuple的元素可以是不同的类型。可以使用std::make_tuple()函数创建一个tuple对象,也可以使用std::get()函数获取tuple对象中的元素。以下是一个创建和使用tuple的例子:
```c++
#include <iostream>
#include <tuple>
#include <string>
int main() {
// 创建一个tuple对象
std::tuple<int, std::string, double> mytuple(10, "hello", 3.14);
// 获取tuple对象中的元素
int myint = std::get<0>(mytuple);
std::string mystr = std::get<1>(mytuple);
double mydouble = std::get<2>(mytuple);
// 输出元素
std::cout << "myint = " << myint << std::endl;
std::cout << "mystr = " << mystr << std::endl;
std::cout << "mydouble = " << mydouble << std::endl;
// 修改元素
std::get<0>(mytuple) = 20;
std::get<1>(mytuple) = "world";
std::get<2>(mytuple) = 6.28;
// 输出修改后的元素
std::cout << "myint = " << std::get<0>(mytuple) << std::endl;
std::cout << "mystr = " << std::get<1>(mytuple) << std::endl;
std::cout << "mydouble = " << std::get<2>(mytuple) << std::endl;
return 0;
}
```
输出结果为:
```
myint = 10
mystr = hello
mydouble = 3.14
myint = 20
mystr = world
mydouble = 6.28
```
相关推荐
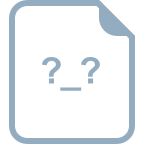













