使用java读取大小为40G的txt文件,并对其中的数据进行降序排序,然后输出到指定txt文件
时间: 2024-05-16 20:17:56 浏览: 83
首先,读取40G的txt文件需要考虑内存限制问题,我们可以采用分块读取的方式,将文件分成多个小块,每次读取一块进行排序,然后输出到指定txt文件中。以下是示例代码:
```java
import java.io.*;
import java.util.*;
public class LargeFileSort {
private static final int MAX_CHUNK_SIZE = 1000000; // 每块最大行数
public static void main(String[] args) throws IOException {
File inputFile = new File("input.txt"); // 待排序的文件
File outputFile = new File("output.txt"); // 输出文件
// 分块读取文件,并对每块数据进行排序
List<File> chunks = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(inputFile), 1024 * 1024)) {
String line;
List<String> lines = new ArrayList<>();
while ((line = reader.readLine()) != null) {
lines.add(line);
if (lines.size() >= MAX_CHUNK_SIZE) {
sortAndSaveChunk(lines);
lines.clear();
}
}
if (!lines.isEmpty()) {
sortAndSaveChunk(lines);
}
}
// 合并排序后的块并输出到指定文件
mergeChunks(chunks, outputFile);
}
private static void sortAndSaveChunk(List<String> lines) throws IOException {
Collections.sort(lines, Collections.reverseOrder()); // 降序排序
File chunk = File.createTempFile("chunk", ".txt");
try (BufferedWriter writer = new BufferedWriter(new FileWriter(chunk))) {
for (String line : lines) {
writer.write(line);
writer.newLine();
}
}
chunks.add(chunk);
}
private static void mergeChunks(List<File> chunks, File outputFile) throws IOException {
PriorityQueue<BufferedReader> heap = new PriorityQueue<>(Comparator.comparing(s -> s.readLine()));
for (File chunk : chunks) {
BufferedReader reader = new BufferedReader(new FileReader(chunk), 1024 * 1024);
heap.offer(reader);
}
try (BufferedWriter writer = new BufferedWriter(new FileWriter(outputFile))) {
while (!heap.isEmpty()) {
BufferedReader reader = heap.poll();
String line = reader.readLine();
if (line != null) {
writer.write(line);
writer.newLine();
heap.offer(reader);
} else {
reader.close();
}
}
}
}
}
```
说明:
- `MAX_CHUNK_SIZE`表示每块最大行数,可以根据实际情况调整。
- `sortAndSaveChunk`方法用于对一块数据进行排序并保存到临时文件中。
- `mergeChunks`方法用于将所有排序后的块合并到输出文件中,采用归并排序的思想,使用优先队列(最小堆)来实现。
- 为了避免内存泄漏,需要在读取完每个块后关闭相关的流并删除临时文件。
阅读全文
相关推荐
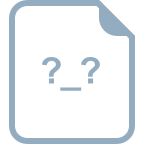
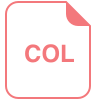
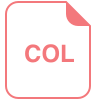
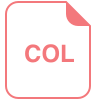
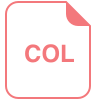
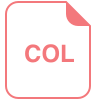
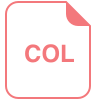
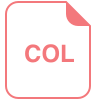
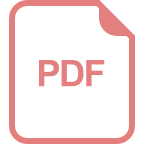
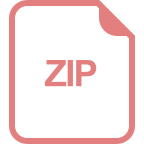
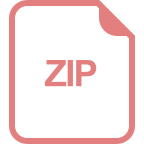
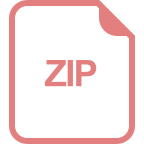
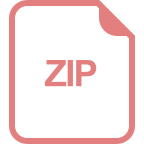