#include<stdio.h> #include<assert.h> #include<mpi.h> #define intT long double f(double x){ return x * x; } double trap(double left_endpt, double right_endpt, int trap_count, double base_len){ double estimate, x; estimate = (f(left_endpt) + f(right_endpt)) / 2.0; for(intT i=1; i< trap_count; i++){ x = left_endpt + i * base_len; estimate += f(x); } estimate = estimate * base_len; return estimate; } int main(void) { int my_rank, comm_sz; intT n = 300000000, local_n; double a = 0.0, b = 2.0, h, local_a, local_b; double local_int, total_int; MPI_Init(NULL, NULL); MPI_Comm_rank(MPI_COMM_WORLD, &my_rank); MPI_Comm_size(MPI_COMM_WORLD, &comm_sz); assert(n % comm_sz == 0); h = (b - a) / n; local_n = n / comm_sz; local_a = a + my_rank * local_n * h; local_b = local_a + local_n * h; local_int = trap(local_a, local_b, local_n, h); MPI_Reduce(&local_int, &total_int, 1, MPI_DOUBLE, MPI_SUM, 0, MPI_COMM_WORLD); if(my_rank == 0){ printf("With a = %lf, b = %lf, n = %ld, total_int = %.10lf\n", a, b, n, total_int); } MPI_Finalize(); return 0; }将此MPI梯形积分程序修改为Pthread梯形积分程序
时间: 2023-11-27 08:55:28 浏览: 160
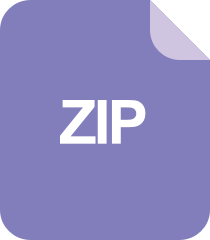
SeqList.h #include <stdio.h> //包含标准输入输出流的头文件 #include <assert.h
以下是将 MPI 梯形积分程序修改为 Pthread 梯形积分程序的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <assert.h>
#define intT long
#define MAX_THREADS 1024
double f(double x) {
return x * x;
}
double trap(double left_endpt, double right_endpt, intT trap_count, double base_len) {
double estimate, x;
estimate = (f(left_endpt) + f(right_endpt)) / 2.0;
for (intT i = 1; i < trap_count; i++) {
x = left_endpt + i * base_len;
estimate += f(x);
}
estimate *= base_len;
return estimate;
}
void* calculate_trap(void* arg) {
intT my_rank = *((intT*) arg);
double a = 0.0, b = 2.0, h, local_a, local_b;
double local_int = 0.0, total_int = 0.0;
intT n = 300000000, local_n;
assert(n % MAX_THREADS == 0);
h = (b - a) / n;
local_n = n / MAX_THREADS;
local_a = a + my_rank * local_n * h;
local_b = local_a + local_n * h;
local_int = trap(local_a, local_b, local_n, h);
total_int += local_int;
return (void*) total_int;
}
int main(void) {
pthread_t threads[MAX_THREADS];
intT thread_args[MAX_THREADS];
void* thread_return[MAX_THREADS];
double total_int = 0.0;
for (intT i = 0; i < MAX_THREADS; i++) {
thread_args[i] = i;
pthread_create(&threads[i], NULL, calculate_trap, (void*) &thread_args[i]);
}
for (intT i = 0; i < MAX_THREADS; i++) {
pthread_join(threads[i], &thread_return[i]);
total_int += *((double*) thread_return[i]);
}
printf("With a = %lf, b = %lf, n = %ld, total_int = %.10lf\n", 0.0, 2.0, 300000000, total_int);
return 0;
}
```
在这个代码中,我们创建了 `MAX_THREADS` 个线程,并且每个线程都会计算一部分积分值,最后将所有线程的积分值求和得到最终的结果。由于 Pthread 不像 MPI 那样需要初始化和结束通信,因此代码更加简洁。
阅读全文
相关推荐
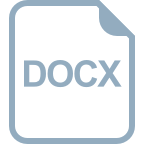
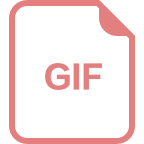
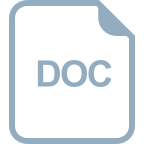
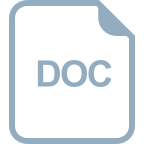
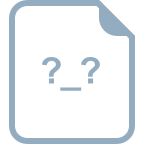
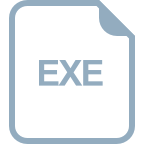
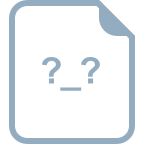
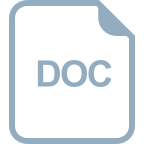
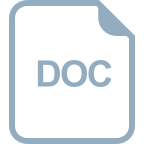
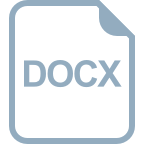
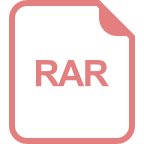
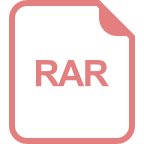