springboot +thymeleaf 用户登陆功能代码
时间: 2023-12-09 20:03:41 浏览: 59
好的,下面是一个简单的使用SpringBoot和Thymeleaf实现用户登录功能的示例代码:
1. 首先,我们需要在pom.xml文件中添加以下依赖:
```xml
<!-- SpringBoot Web依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Thymeleaf 模板引擎依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- SpringBoot 数据库依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- H2 内存数据库依赖 -->
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
```
2. 然后,我们需要创建一个User实体类,用于表示用户信息:
```java
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String username;
@Column(nullable = false)
private String password;
// Getters and Setters
}
```
3. 接下来,我们需要创建一个UserRepository接口,用于操作用户数据:
```java
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
```
4. 然后,我们需要创建一个UserController类,用于处理用户登录请求:
```java
@Controller
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/login")
public String login(Model model) {
model.addAttribute("user", new User());
return "login";
}
@PostMapping("/login")
public String login(@ModelAttribute("user") User user, HttpSession session) {
User dbUser = userRepository.findByUsername(user.getUsername());
if (dbUser != null && dbUser.getPassword().equals(user.getPassword())) {
session.setAttribute("user", dbUser);
return "redirect:/";
}
return "login";
}
@GetMapping("/logout")
public String logout(HttpSession session) {
session.removeAttribute("user");
return "redirect:/login";
}
}
```
5. 最后,我们需要创建一个login.html文件,用于展示登录表单:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form th:action="@{/login}" th:object="${user}" method="post">
<div>
<label>Username:</label>
<input type="text" th:field="*{username}" />
</div>
<div>
<label>Password:</label>
<input type="password" th:field="*{password}" />
</div>
<div>
<button type="submit">Login</button>
</div>
</form>
</body>
</html>
```
以上就是一个简单的使用SpringBoot和Thymeleaf实现用户登录功能的示例代码,希望能对您有所帮助。
相关推荐
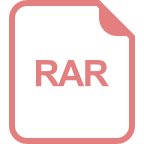
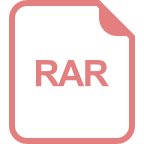
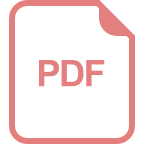














