用c++实现小车倒立摆控制
时间: 2023-08-24 10:50:36 浏览: 58
小车倒立摆控制是一个经典的控制问题,它涉及到小车的运动学和倒立摆的动力学,需要用到控制算法来实现稳定控制。下面是一个简单的C++程序,演示如何实现小车倒立摆控制:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 定义小车倒立摆的状态变量
double theta, theta_dot, x, x_dot;
// 定义控制器参数
double Kp_theta = 100, Ki_theta = 0.1, Kd_theta = 20;
double Kp_x = 10, Ki_x = 0.1, Kd_x = 2;
// 定义控制器误差变量
double error_theta = 0, error_theta_prev = 0, error_theta_int = 0;
double error_x = 0, error_x_prev = 0, error_x_int = 0;
// 计算控制力矩
void control(double setpoint_theta, double setpoint_x, double &f, double &u)
{
// 计算角度误差
error_theta = setpoint_theta - theta;
// 计算角度误差积分
error_theta_int += error_theta;
// 计算角度误差变化率
double error_theta_diff = error_theta - error_theta_prev;
// 计算角度控制力矩
f = Kp_theta * error_theta + Ki_theta * error_theta_int + Kd_theta * error_theta_diff;
// 保存角度误差值
error_theta_prev = error_theta;
// 计算位置误差
error_x = setpoint_x - x;
// 计算位置误差积分
error_x_int += error_x;
// 计算位置误差变化率
double error_x_diff = error_x - error_x_prev;
// 计算位置控制力矩
u = Kp_x * error_x + Ki_x * error_x_int + Kd_x * error_x_diff;
// 保存位置误差值
error_x_prev = error_x;
}
// 模拟小车倒立摆运动
void simulate(double setpoint_theta, double setpoint_x)
{
// 模拟时间步长
double dt = 0.01;
// 模拟时间
double t = 0;
// 模拟主循环
while (t < 10)
{
// 计算控制力矩
double f, u;
control(setpoint_theta, setpoint_x, f, u);
// 计算小车倒立摆状态变化率
double theta_ddot = 9.8 / 0.5 * sin(theta) + f / 0.5 * cos(theta);
double x_ddot = u / 1 - 0.5 * theta_dot * theta_dot * sin(theta);
// 更新小车倒立摆状态
theta_dot += theta_ddot * dt;
theta += theta_dot * dt;
x_dot += x_ddot * dt;
x += x_dot * dt;
// 输出小车倒立摆状态
cout << t << "\t" << theta << "\t" << x << endl;
// 更新时间
t += dt;
}
}
int main()
{
// 设置目标状态
double setpoint_theta = 0;
double setpoint_x = 0;
// 模拟小车倒立摆运动
simulate(setpoint_theta, setpoint_x);
return 0;
}
```
这段代码实现了一个简单的PID控制器,来控制小车倒立摆保持直立。在模拟主循环中,它计算控制力矩,根据控制力矩更新小车倒立摆状态,并输出小车倒立摆状态。你可以根据需要修改控制器参数和目标状态,来实现不同的控制任务。
相关推荐
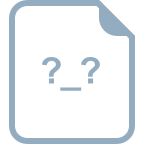














