用c语言写一个程序由用户输入字符串。编辑程序将其中大写字符取出放入数组c中,利用指针完成。
时间: 2023-06-12 21:07:42 浏览: 120
以下是用 C 语言实现的程序,可以实现由用户输入字符串,将其中大写字符取出并放入数组 c 中:
```c
#include <stdio.h>
#include <ctype.h>
#define MAX_LEN 100
int main() {
char str[MAX_LEN + 1];
char c[MAX_LEN + 1];
char *p = c;
int i, j = 0;
printf("请输入字符串:");
fgets(str, sizeof(str), stdin);
for (i = 0; str[i] != '\0'; i++) {
if (isupper(str[i])) {
*(p++) = str[i];
j++;
}
}
printf("大写字符个数:%d\n", j);
printf("大写字符为:");
for (i = 0; i < j; i++) {
printf("%c ", c[i]);
}
printf("\n");
return 0;
}
```
程序中使用了 `isupper()` 函数判断一个字符是否为大写字符,然后将大写字符存入数组 c 中,最后输出大写字符的个数和大写字符。指针变量 p 指向数组 c 的首地址,每存入一个字符,指针 p 向后移动一位,保证每次存入的字符都存入数组 c 的下一个位置。
相关问题
用c语言做一个程序,输入一个字符串,将这个字符串中的大写英文字母除去,输出剩余的字符组成的字符串。(用指针做)输入形式:输入一个字符串,可能包含有大写英文字母。输出形式:输出除去大写英文字母的剩余字符串。
下面是用指针实现的 C 语言程序代码:
```c
#include <stdio.h>
void remove_uppercase(char *str) {
char *p = str;
while (*p != '\0') {
if (*p >= 'A' && *p <= 'Z') {
char *q = p;
while (*q != '\0') {
*q = *(q + 1);
q++;
}
} else {
p++;
}
}
}
int main() {
char str[100];
printf("请输入一个字符串:");
scanf("%s", str);
remove_uppercase(str);
printf("除去大写字母后的字符串是:%s\n", str);
return 0;
}
```
程序中的 `remove_uppercase` 函数用于去除输入字符串中的大写英文字母。它采用两个指针 `p` 和 `q`,其中 `p` 用于遍历字符串中的每个字符,如果当前字符是大写英文字母,就用 `q` 指针从当前位置开始,将后面的字符依次往前移动一个位置,最终将这个字符删除。如果当前字符不是大写英文字母,就继续遍历下一个字符。
使用c语言,利用指针编程输入一个字符串,找出其中的大写字母、小写字母,空格,数字,及其他字符的个数。
以下是使用C语言,利用指针编程输入一个字符串,找出其中的大写字母、小写字母,空格,数字,及其他字符的个数的代码:
```c
#include <stdio.h>
#include <stdlib.h>
void count(char *str, int *num) {
while (*str != '\0') {
if (*str >= 'A' && *str <= 'Z') {
num[0]++;
} else if (*str >= 'a' && *str <= 'z') {
num[1]++;
} else if (*str == ' ' || *str == '\t') {
num[2]++;
} else if (*str >= '0' && *str <= '9') {
num[3]++;
} else {
num[4]++;
}
str++;
}
}
int main() {
char str[100];
int num[5] = {0};
printf("请输入一个字符串:");
gets(str);
count(str, num);
printf("大写字母:%d\n", num[0]); printf("小写字母:%d\n", num[1]);
printf("空格:%d\n", num[2]);
printf("数字:%d\n", num[3]);
printf("其他字符:%d\n", num[4]);
return 0;
}
```
阅读全文
相关推荐
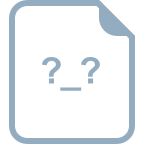













