qt中QAbstractItemModel的insertrow函数如何实现,可以写一个用C++实现的实例吗
时间: 2024-03-09 10:47:25 浏览: 118
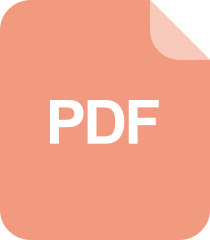
table对象中的insertRow与deleteRow使用示例
QAbstractItemModel的insertRow()函数用于在指定位置插入一行数据,其函数声明如下:
```
bool insertRow(int row, const QModelIndex &parent = QModelIndex());
```
其中,row参数指定新行的插入位置,parent参数指定新行的父项索引,默认值为无效索引QModelIndex(),表示新行是模型的根节点。
下面是一个简单的C++实现示例,实现了一个自定义的QAbstractItemModel子类MyModel,提供了insertRow()函数,用于向模型中插入新行:
```
class MyModel : public QAbstractItemModel
{
public:
MyModel(QObject *parent = nullptr);
// 重写QAbstractItemModel的虚函数
QModelIndex index(int row, int column, const QModelIndex &parent = QModelIndex()) const override;
QModelIndex parent(const QModelIndex &child) const override;
int rowCount(const QModelIndex &parent = QModelIndex()) const override;
int columnCount(const QModelIndex &parent = QModelIndex()) const override;
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override;
// 自定义函数
bool insertRow(int row, const QModelIndex &parent = QModelIndex());
private:
QList<QStringList> m_data; // 模型数据
};
MyModel::MyModel(QObject *parent)
: QAbstractItemModel(parent)
{
QStringList header;
header << "Name" << "Age" << "Gender";
m_data.append(header);
QStringList row1;
row1 << "Alice" << "25" << "Female";
m_data.append(row1);
QStringList row2;
row2 << "Bob" << "30" << "Male";
m_data.append(row2);
}
QModelIndex MyModel::index(int row, int column, const QModelIndex &parent) const
{
if (!hasIndex(row, column, parent))
return QModelIndex();
if (!parent.isValid())
return createIndex(row, column);
else
return createIndex(row, column, parent.row() * columnCount() + parent.column() + 1);
}
QModelIndex MyModel::parent(const QModelIndex &child) const
{
if (!child.isValid())
return QModelIndex();
int idx = child.internalId() - 1;
if (idx < 0)
return QModelIndex();
else
return createIndex(idx / columnCount(), idx % columnCount());
}
int MyModel::rowCount(const QModelIndex &parent) const
{
if (!parent.isValid())
return m_data.size();
else
return 0;
}
int MyModel::columnCount(const QModelIndex &parent) const
{
if (!parent.isValid())
return m_data.isEmpty() ? 0 : m_data.first().size();
else
return 0;
}
QVariant MyModel::data(const QModelIndex &index, int role) const
{
if (!index.isValid())
return QVariant();
if (role == Qt::DisplayRole || role == Qt::EditRole)
return m_data.at(index.row() + 1).at(index.column());
else
return QVariant();
}
bool MyModel::insertRow(int row, const QModelIndex &parent)
{
beginInsertRows(parent, row, row);
QStringList newRow;
for (int i = 0; i < columnCount(parent); ++i) {
newRow << "";
}
m_data.insert(row + 1, newRow);
endInsertRows();
return true;
}
```
在使用时,可以先实例化一个MyModel对象,然后调用其insertRow()函数插入新行:
```
MyModel *model = new MyModel();
model->insertRow(1); // 在第2行插入新行
```
这样就可以在第2行插入一行空数据了。
阅读全文
相关推荐
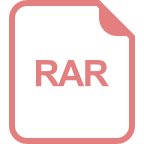
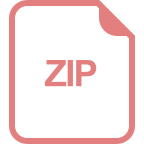















