python统计字符串出现次数
时间: 2023-11-10 20:06:34 浏览: 93
你可以使用 Python 中的 `count()` 方法来统计字符串中某个字符或子字符串出现的次数。例如,给定一个字符串 `s`,统计其中字符 `a` 出现的次数,可以使用以下代码:
```python
s = "hello world"
count = s.count("l")
print(count) # 输出 3
```
如果要统计子字符串出现的次数,可以将子字符串作为参数传递给 `count()` 方法。例如,给定一个字符串 `s`,统计其中子字符串 `"lo"` 出现的次数,可以使用以下代码:
```python
s = "hello world"
count = s.count("lo")
print(count) # 输出 1
```
如果要忽略大小写进行统计,可以先将字符串转换为小写或大写形式,然后再调用 `count()` 方法。例如,给定一个字符串 `s`,统计其中字符 `o` 出现的次数(忽略大小写),可以使用以下代码:
```python
s = "hello world"
s_lower = s.lower() # 将字符串转换为小写形式
count = s_lower.count("o")
print(count) # 输出 2
```
相关问题
python统计字符串出现的次数
你可以使用 Python 中的 `count()` 函数来统计字符串中某个子字符串出现的次数。
例如,假设你想要统计字符串 `"hello world"` 中字母 `"l"` 出现的次数,你可以使用以下代码:
```python
string = "hello world"
count = string.count("l")
print(count) # 输出 3
```
注意,`count()` 函数区分字母大小写,因此如果你想要统计字符串中某个子字符串不区分大小写出现的次数,你需要先将字符串全部转换为小写或大写字母,然后再调用 `count()` 函数。例如:
```python
string = "HeLLo WoRLd"
count = string.lower().count("l")
print(count) # 输出 3
```
这里先使用 `lower()` 函数将字符串转换为小写字母,然后再统计字母 `"l"` 出现的次数。
python字典统计字符串出现次数
可以使用python的字典来统计字符串中每个字符出现的次数。
例如,假设有一个字符串s:
```python
s = "hello world"
```
我们可以使用一个字典来统计每个字符出现的次数:
```python
count = {}
for char in s:
if char in count:
count[char] += 1
else:
count[char] = 1
```
最终得到的字典count将包含每个字符出现的次数:
```python
{'h': 1, 'e': 1, 'l': 3, 'o': 2, ' ': 1, 'w': 1, 'r': 1, 'd': 1}
```
我们可以使用dict.items()方法来遍历字典,并输出每个字符出现的次数:
```python
for char, count in count.items():
print(char, count)
```
输出为:
```
h 1
e 1
l 3
o 2
1
w 1
r 1
d 1
```
阅读全文
相关推荐
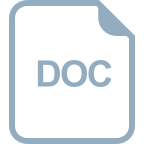
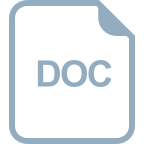
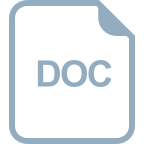
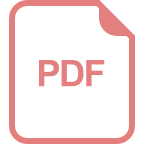











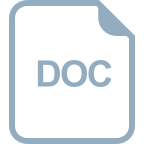