vue判断一个数组对象中是否有相同数据
时间: 2024-05-06 21:17:16 浏览: 134
可以通过遍历数组对象,逐个比较每个元素是否相同来判断。不过这种方法效率比较低,当数组元素比较多时会耗费大量时间。另外,还可以使用ES6的Set对象来判断,因为Set对象中不允许有重复的元素,可以把数组对象转换成Set对象,然后判断两者长度是否一致,如果一致则表示没有重复元素,反之则有重复元素。
下面是代码示例:
```javascript
// 遍历数组对象,逐个比较每个元素是否相同
function hasRepeat(arr) {
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (JSON.stringify(arr[i]) === JSON.stringify(arr[j])) {
return true;
}
}
}
return false;
}
// 使用Set对象来判断
function hasRepeat2(arr) {
return arr.length !== new Set(arr.map(JSON.stringify)).size;
}
```
其中,`JSON.stringify()`方法可以将对象转换成字符串,便于比较。
相关问题
vue.js 根据标识判断两个数组对象,分别取到相同和不同数据
Vue.js 是一个流行的前端框架,它可以帮助你构建用户界面,并提供响应式的数据绑定功能。当你需要比较两个数组对象并找出相同和不同的元素时,可以利用 JavaScript 的 `filter()` 和 `includes()` 或者一些辅助库如 Vue computed properties 或者 lodash 中的函数。
首先,你可以创建一个计算属性(computed property),比如 `findSameAndDifferent`,来实现这个功能:
```javascript
<template>
<div>
<!-- 其他模板内容 -->
<p>相同元素: {{ sameElements }}</p>
<p>不同元素: {{ differentElements }}</p>
</div>
</template>
<script>
import { mapGetters } from 'vuex';
export default {
computed: {
...mapGetters(['arrayA', 'arrayB']), // 如果在 vuex 中获取数组
findSameAndDifferent() {
const sameElements = this.arrayA.filter(obj => this.arrayB.includes(obj.id));
const differentElementsArrayA = this.arrayA.filter(obj => !this.arrayB.includes(obj.id));
const differentElementsArrayB = this.arrayB.filter(obj => !this.arrayA.includes(obj.id));
return {
sameElements,
differentElements: {
arrayA: differentElementsArrayA,
arrayB: differentElementsArrayB
}
};
}
}
};
</script>
```
在这个例子中,我们先通过 `filter()` 函数找出在 `arrayB` 中存在 id 对应项的元素,作为相同元素。然后,分别从每个原始数组中过滤出不在对方中存在的元素,作为不同元素。
在vue :class中运用方法查询数组对象中,所有数据中一个数据的和另一个数据相同
可以使用计算属性来查询数组对象中所有数据中一个数据的和另一个数据相同的项,然后在:class中使用这个计算属性来动态绑定样式。
下面是一个示例:
```
<template>
<div>
<div v-for="(item, index) in items" :key="index" :class="getClass(item)">
{{ item.name }} - {{ item.value1 }} - {{ item.value2 }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
items: [
{ name: 'item1', value1: 5, value2: 5 },
{ name: 'item2', value1: 10, value2: 5 },
{ name: 'item3', value1: 15, value2: 5 },
{ name: 'item4', value1: 20, value2: 10 },
{ name: 'item5', value1: 25, value2: 10 },
],
};
},
computed: {
equalValueItems() {
return this.items.filter((item) => item.value1 === item.value2);
},
},
methods: {
getClass(item) {
return this.equalValueItems.includes(item) ? 'equal' : 'not-equal';
},
},
};
</script>
<style>
.equal {
background-color: green;
}
.not-equal {
background-color: red;
}
</style>
```
上面的代码中,我们首先定义了一个数组对象items,包含了5个项,每个项都有两个属性value1和value2。然后我们定义了一个计算属性equalValueItems,用来查询数组对象中所有数据中一个数据的和另一个数据相同的项。
接着我们定义了一个方法getClass,用来判断每个项是否属于equalValueItems数组中的项,如果是,则返回'equal',否则返回'not-equal'。最后在:class中动态绑定样式。
运行上面的代码,我们可以看到相等的项的背景色是绿色的,不相等的项的背景色是红色的。
希望这个示例对你有帮助!
阅读全文
相关推荐
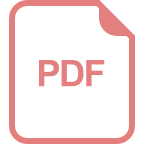
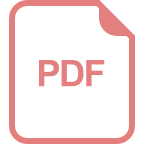
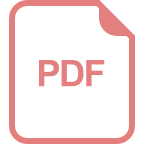













