c语言校园路线查询代码
时间: 2023-08-12 20:20:14 浏览: 101
校园路线查询,可以使用图论中的最短路径算法,比如Dijkstra算法或者Floyd算法,以下是一个简单的使用Dijkstra算法的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
#define MAX_VERTEX_NUM 100 // 最大顶点数
#define INFINITY INT_MAX // 无穷大
typedef int ElemType;
typedef int WeightType;
// 邻接表中的边
typedef struct ArcNode {
int adjvex; // 邻接点在数组中的下标
WeightType weight; // 边的权重
struct ArcNode *nextarc; // 指向下一条边的指针
} ArcNode;
// 邻接表中的顶点
typedef struct VNode {
ElemType data; // 顶点的数据
ArcNode *firstarc; // 指向第一条依附该顶点的边的指针
} VNode;
// 使用邻接表存储图
typedef struct {
VNode vertices[MAX_VERTEX_NUM]; // 顶点数组
int vexnum, arcnum; // 顶点数和边数
} ALGraph;
// Dijkstra算法求最短路径
void Dijkstra(ALGraph *G, int s, int dist[], int path[]) {
int i, j, k, min;
int *visited = (int *)malloc(G->vexnum * sizeof(int)); // 标记数组
for (i = 0; i < G->vexnum; i++) {
visited[i] = 0; // 初始化为未访问
dist[i] = INFINITY; // 初始化为无穷大
path[i] = -1; // 初始化为-1
}
visited[s] = 1; // 标记起点已访问
dist[s] = 0; // 起点到起点的距离为0
path[s] = -1; // 起点没有前驱顶点
ArcNode *p = NULL;
for (i = 0; i < G->vexnum - 1; i++) { // 进行vexnum-1次循环,每次确定一个顶点的最短路径
min = INFINITY;
for (j = 0; j < G->vexnum; j++) { // 选取当前未访问的离起点最近的顶点
if (!visited[j] && dist[j] < min) {
min = dist[j];
k = j;
}
}
visited[k] = 1; // 标记顶点k已访问
p = G->vertices[k].firstarc;
while (p != NULL) { // 更新起点到其他顶点的距离
if (!visited[p->adjvex] && dist[k] + p->weight < dist[p->adjvex]) {
dist[p->adjvex] = dist[k] + p->weight;
path[p->adjvex] = k;
}
p = p->nextarc;
}
}
free(visited);
}
// 创建邻接表
void CreateGraph(ALGraph *G) {
int i, j, k, w;
ArcNode *p = NULL;
printf("请输入顶点数和边数:");
scanf("%d%d", &(G->vexnum), &(G->arcnum));
printf("请输入各个顶点的数据:");
for (i = 0; i < G->vexnum; i++) {
scanf("%d", &(G->vertices[i].data));
G->vertices[i].firstarc = NULL;
}
printf("请输入每条边的起点、终点和权重:\n");
for (k = 0; k < G->arcnum; k++) {
scanf("%d%d%d", &i, &j, &w);
p = (ArcNode *)malloc(sizeof(ArcNode));
p->adjvex = j;
p->weight = w;
p->nextarc = G->vertices[i].firstarc;
G->vertices[i].firstarc = p;
}
}
int main() {
ALGraph G;
int s, t, i, j, len;
int dist[MAX_VERTEX_NUM], path[MAX_VERTEX_NUM];
CreateGraph(&G);
printf("请输入起点和终点的下标:");
scanf("%d%d", &s, &t);
Dijkstra(&G, s, dist, path);
printf("从%d到%d的最短路径为:", s, t);
len = 0;
i = t;
while (i != -1) { // 从终点依次输出路径上的顶点
j = path[i];
if (j != -1) {
printf("%d->", G.vertices[i].data);
len += dist[i];
} else {
printf("%d", G.vertices[i].data);
}
i = j;
}
printf("\n路径长度为:%d\n", len);
return 0;
}
```
该代码使用邻接表存储图,使用Dijkstra算法求最短路径,可以根据输入的起点和终点下标输出最短路径和路径长度。
阅读全文
相关推荐











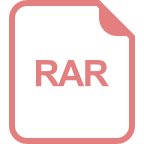





