java字符串转多层集合
时间: 2023-05-04 10:02:17 浏览: 68
Java字符串转多层集合可以用递归算法来实现。首先需要将字符串根据分隔符切割成一个数组,然后判断该数组首元素是否是一个集合对象,如果是则递归处理该集合对象中的元素,否则直接将该元素添加到上一层集合中。在处理最内层集合时,需要将其中的元素转换为对应的数据类型,例如将字符串"1"转换为整型1。最后返回最外层的集合即可。
以下是一个示例代码:
```java
import java.util.*;
public class StringToCollection {
public static void main(String[] args) {
String str = "[1,2,[3,4,[5,6]],7]";
List<Object> result = (List<Object>)stringToCollection(str);
System.out.println(result);
}
public static Object stringToCollection(String str) {
char firstChar = str.charAt(0);
char lastChar = str.charAt(str.length() - 1);
if (firstChar != '[' || lastChar != ']') {
// 非集合字符串
if (str.matches("-?\\d+")) {
// 整型
return Integer.parseInt(str);
} else {
// 字符串
return str;
}
}
// 集合字符串
List<Object> list = new ArrayList<>();
int startIndex = 1;
int endIndex = 1;
int level = 0;
for (int i = 1; i < str.length() - 1; i++) {
char ch = str.charAt(i);
if (ch == '[') {
level++;
} else if (ch == ']') {
level--;
} else if (ch == ',' && level == 0) {
endIndex = i;
list.add(stringToCollection(str.substring(startIndex, endIndex)));
startIndex = i + 1;
}
}
list.add(stringToCollection(str.substring(startIndex, str.length() - 1)));
return list;
}
}
```
使用该代码,可以将字符串"[1,2,[3,4,[5,6]],7]"转换为如下的多层集合:
```
[1, 2, [3, 4, [5, 6]], 7]
```
相关推荐
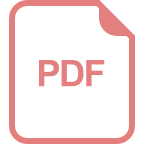








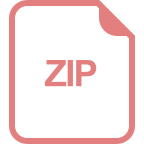
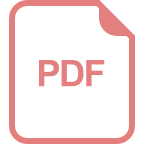
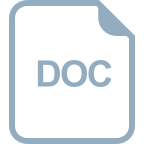
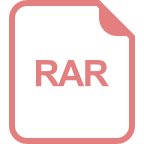
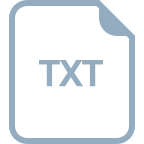
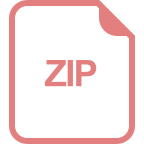