用java写一个愤怒的小鸟
时间: 2023-09-10 07:13:46 浏览: 98
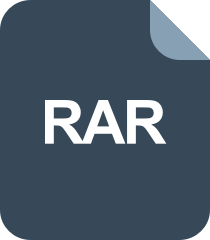
基于Java的愤怒的小鸟游戏的设计与实现【LW文档+PPT+开题报告+数据库+讲解视频】
以下是一个简单的用 Java 编写愤怒的小鸟的示例代码,用到了JavaFX和动画效果:
```java
import javafx.animation.Animation;
import javafx.animation.KeyFrame;
import javafx.animation.KeyValue;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.geometry.Bounds;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.input.MouseEvent;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import javafx.util.Duration;
public class AngryBirds extends Application {
private ImageView bird;
private Circle target;
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// 创建鸟和目标的对象
bird = new ImageView(new Image("bird.png"));
bird.setFitWidth(50);
bird.setFitHeight(50);
target = new Circle(25);
target.setFill(Color.RED);
// 绑定鼠标点击事件
bird.setOnMouseClicked((MouseEvent event) -> {
shoot();
});
// 创建场景
Group root = new Group();
Scene scene = new Scene(root, 640, 480);
Pane pane = new Pane();
pane.getChildren().addAll(bird, target);
root.getChildren().add(pane);
primaryStage.setScene(scene);
primaryStage.show();
}
private void shoot() {
// 计算目标的位置
Bounds birdBounds = bird.getBoundsInParent();
double targetX = birdBounds.getMaxX() + 50;
double targetY = birdBounds.getMinY() + birdBounds.getHeight() / 2;
// 创建目标的动画
Timeline timeline = new Timeline();
timeline.setCycleCount(1);
timeline.setAutoReverse(false);
KeyValue kvX = new KeyValue(target.centerXProperty(), targetX);
KeyValue kvY = new KeyValue(target.centerYProperty(), targetY);
KeyFrame kf = new KeyFrame(Duration.seconds(2), kvX, kvY);
timeline.getKeyFrames().add(kf);
timeline.setOnFinished((event) -> {
// 动画结束后检查是否命中目标
if (bird.getBoundsInParent().intersects(target.getBoundsInParent())) {
System.out.println("命中目标!");
} else {
System.out.println("未命中目标。");
}
});
timeline.play();
}
}
```
这个小程序创建了一个场景,里面包含了一个鸟和一个目标,当点击鸟时,鸟会飞向目标并做出动画效果,动画结束后检查是否命中目标。这个程序只是一个简单的示例,你可以根据需求进行修改和扩展。
阅读全文
相关推荐
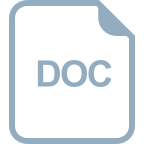
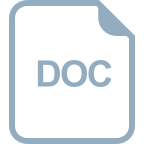
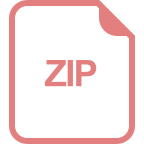
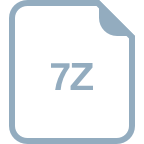
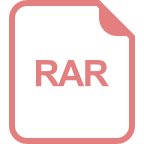
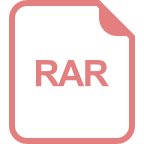






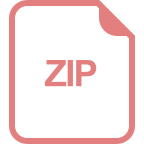
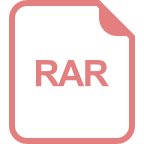
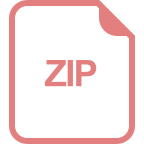
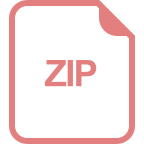
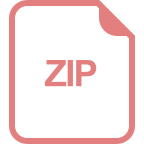
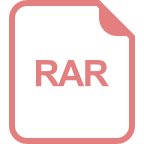