java 通过两个参数:图片名与指定系统名,在多个系统的指定文件夹下查找图片并存入到数据库中
时间: 2024-03-22 12:40:02 浏览: 54
好的,我明白您的问题了。首先需要确定您使用的是什么数据库,并且需要编写一个 Java 程序来实现您的需求。下面是实现该功能的基本步骤:
1. 获取图片名和指定系统名作为输入参数。
2. 根据指定系统名确定图片所在的文件夹。
3. 在该文件夹下查找指定名称的图片文件。
4. 如果找到图片文件,则读取图片内容,并将其存入数据库中。
5. 如果没有找到图片文件,则记录日志并提示用户。
下面是一个简单的 Java 程序示例,可以帮助您实现上述步骤:
```java
import java.io.File;
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
public class ImageLoader {
public static void main(String[] args) {
try {
// 获取输入参数
String imageName = args[0];
String systemName = args[1];
// 确定文件夹路径
String folderPath = "C:\\" + systemName + "\\images\\";
// 查找文件
File imageFile = new File(folderPath + imageName);
if (!imageFile.exists()) {
System.out.println("Image not found.");
return;
}
// 读取文件内容
FileInputStream fis = new FileInputStream(imageFile);
byte[] imageData = new byte[(int) imageFile.length()];
fis.read(imageData);
fis.close();
// 存入数据库
Class.forName("com.mysql.jdbc.Driver");
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
String sql = "INSERT INTO images (name, data) VALUES (?, ?)";
PreparedStatement ps = con.prepareStatement(sql);
ps.setString(1, imageName);
ps.setBytes(2, imageData);
ps.executeUpdate();
ps.close();
con.close();
System.out.println("Image saved successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
需要注意的是,上述代码仅作为示例,您需要根据自己的具体情况进行修改和完善。同时,还需要在程序运行前确保数据库已经正确配置,并且需要提前下载并导入 MySQL 驱动程序。
阅读全文
相关推荐

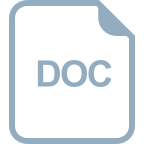
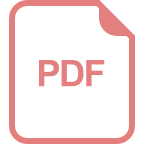
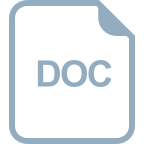
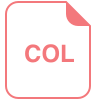
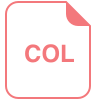
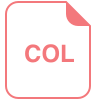
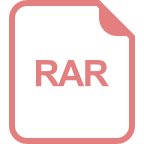
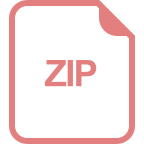
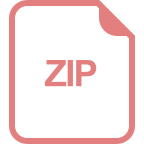
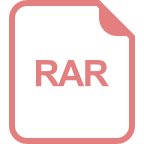