如何使用idea在名为jdbc的数据库中创建数据表user
时间: 2024-03-27 20:40:52 浏览: 216
在 IDEA 中使用 JDBC 创建数据库表和实现增删改查等操作需要以下步骤:
1. 配置数据库连接
首先需要在 IDEA 中配置数据库连接,可以通过菜单栏的 "View" -> "Tool Windows" -> "Database" 打开数据库工具窗口,然后在窗口中点击加号按钮,选择 "Data source" -> "MySQL",填写数据库连接信息,包括数据库地址、端口号、用户名和密码等。
2. 创建 JavaBean 类
创建一个名为 User 的 JavaBean 类,表示要在数据库中创建的数据表。
```java
public class User {
private int id;
private String name;
private String password;
private int age;
// getter 和 setter 方法省略
}
```
3. 创建数据表
在 IDEA 中使用 JDBC 创建数据表需要编写 SQL 语句,可以使用以下代码创建名为 user 的数据表:
```java
Connection conn = null;
Statement stmt = null;
try {
conn = dataSource.getConnection(); // 获取数据库连接
stmt = conn.createStatement(); // 创建 Statement 对象
String sql = "CREATE TABLE IF NOT EXISTS user ("
+ "id INT(11) NOT NULL AUTO_INCREMENT,"
+ "name VARCHAR(50) NOT NULL,"
+ "password VARCHAR(50) NOT NULL,"
+ "age INT(11),"
+ "PRIMARY KEY (id))";
stmt.executeUpdate(sql); // 执行 SQL 语句
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) {
stmt.close(); // 关闭 Statement 对象
}
if (conn != null) {
conn.close(); // 关闭数据库连接
}
} catch (SQLException e) {
e.printStackTrace();
}
}
```
以上代码中,首先通过 dataSource.getConnection() 方法获取数据库连接,然后创建 Statement 对象并编写 SQL 语句,使用 executeUpdate() 方法执行 SQL 语句创建数据表。最后需要在 finally 块中关闭 Statement 对象和数据库连接。
4. 测试创建数据表
可以使用以下代码测试是否成功创建数据表:
```java
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
conn = dataSource.getConnection(); // 获取数据库连接
stmt = conn.createStatement(); // 创建 Statement 对象
String sql = "SELECT * FROM user";
rs = stmt.executeQuery(sql); // 执行查询语句
ResultSetMetaData metaData = rs.getMetaData(); // 获取结果集元数据
int columnCount = metaData.getColumnCount(); // 获取结果集列数
for (int i = 1; i <= columnCount; i++) {
System.out.print(metaData.getColumnName(i) + "\t"); // 输出列名
}
System.out.println();
while (rs.next()) {
System.out.print(rs.getInt("id") + "\t");
System.out.print(rs.getString("name") + "\t");
System.out.print(rs.getString("password") + "\t");
System.out.print(rs.getInt("age") + "\t");
System.out.println();
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close(); // 关闭 ResultSet 对象
}
if (stmt != null) {
stmt.close(); // 关闭 Statement 对象
}
if (conn != null) {
conn.close(); // 关闭数据库连接
}
} catch (SQLException e) {
e.printStackTrace();
}
}
```
以上代码中,首先执行查询语句获取数据表的所有记录,然后使用 ResultSetMetaData 获取结果集的元数据,输出列名和每条记录的具体值。如果执行成功,则说明已经成功创建了名为 user 的数据表。
阅读全文
相关推荐
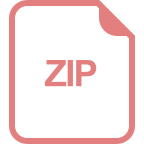
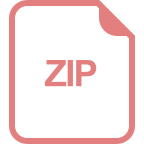
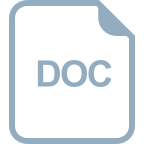
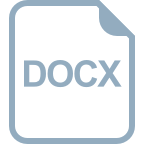
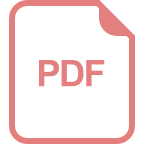
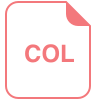
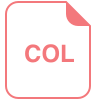
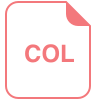










