用C语言建立两个递增有序单向链表,合并为一个递增有序链表
时间: 2024-06-14 22:05:19 浏览: 204
以下是用C语言建立两个递增有序单向链表,并将它们合并为一个递增有序链表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 创建新节点
struct Node* createNode(int value) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 合并两个有序链表
struct Node* mergeLists(struct Node* list1, struct Node* list2) {
// 创建一个新的头节点
struct Node* head = createNode(0);
struct Node* current = head;
// 比较两个链表的节点值,将较小的节点插入到新链表中
while (list1 != NULL && list2 != NULL) {
if (list1->data <= list2->data) {
current->next = list1;
list1 = list1->next;
} else {
current->next = list2;
list2 = list2->next;
}
current = current->next;
}
// 将剩余的节点连接到新链表的末尾
if (list1 != NULL) {
current->next = list1;
} else {
current->next = list2;
}
// 返回新链表的头节点
return head->next;
}
// 打印链表
void printList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
// 创建第一个有序链表
struct Node* list1 = createNode(1);
list1->next = createNode(3);
list1->next->next = createNode(5);
// 创建第二个有序链表
struct Node* list2 = createNode(2);
list2->next = createNode(4);
list2->next->next = createNode(6);
// 合并两个有序链表
struct Node* mergedList = mergeLists(list1, list2);
// 打印合并后的链表
printList(mergedList);
return 0;
}
```
输出结果为:1 2 3 4 5 6
阅读全文
相关推荐




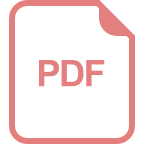
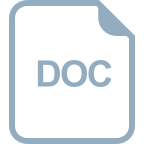
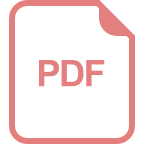
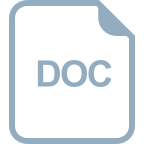
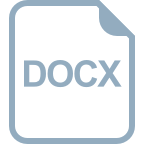
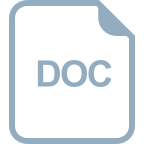
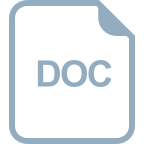
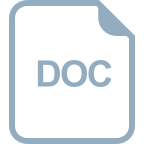
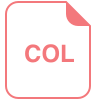
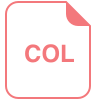
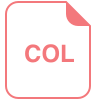
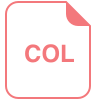