用MASM编写程序,输入一个不小于50的整数n,用递归计算n!,以十进制数输出。
时间: 2024-05-13 14:16:47 浏览: 8
以下是使用MASM汇编语言编写的程序,可以计算n!(n大于等于50)并输出结果。该程序使用递归方式实现阶乘计算。
```
TITLE Recursive Factorial Calculation (MASM Assembly)
; Define constants
COUNT equ 50 ; Minimum value of n
MAX_DIGITS equ 100 ; Maximum number of decimal digits in the result
; Define data section
.DATA
nPrompt db "Enter a value for n (at least 50): ", 0
resultPrompt db "Result is: ", 0
result db MAX_DIGITS dup (0) ; Buffer to hold the result
; Define code section
.CODE
main PROC
; Initialize stack frame
push ebp
mov ebp, esp
; Prompt user for input
mov eax, nPrompt
call WriteString
call ReadInt
; Check input
cmp eax, COUNT
jl error
; Calculate factorial
push eax ; Save n on stack
call factorial
add esp, 4 ; Pop n from stack
; Convert result to decimal
mov esi, result ; esi points to buffer for result
mov ecx, MAX_DIGITS ; ecx = maximum number of digits
call intToStr ; Convert result to string
; Output result
mov eax, resultPrompt
call WriteString
mov eax, result
call WriteString
; Clean up stack and exit
pop ebp
ret
main ENDP
factorial PROC
; Initialize stack frame
push ebp
mov ebp, esp
; Get n from stack
mov eax, [ebp+8]
; Base case: n = 0 or 1
cmp eax, 1
jle done
; Recursive case: n > 1
dec eax ; Decrement n
push eax ; Save n on stack
call factorial ; Calculate (n-1)!
pop eax ; Restore n from stack
imul eax, [ebp+8] ; Multiply n by (n-1)!
jmp done
done:
; Clean up stack and return
pop ebp
ret
factorial ENDP
; Converts an integer in eax to a string in esi
; Assumes esi points to a buffer with enough space for the string
intToStr PROC
; Initialize stack frame
push ebp
mov ebp, esp
; Save registers
push esi
push ebx
; Convert integer to string
mov ebx, 10 ; Base 10
mov ecx, MAX_DIGITS ; Maximum number of digits
mov edx, 0 ; Start with zero remainder
loop:
xor edx, edx ; Clear remainder
div ebx ; Divide by 10
add dl, '0' ; Convert remainder to ASCII digit
mov byte ptr [esi+ecx-1], dl ; Store digit in buffer
dec ecx ; Decrement digit count
test eax, eax ; Check for zero quotient
jnz loop ; Continue if not zero
; Trim leading zeros
mov ecx, MAX_DIGITS ; Maximum number of digits
mov esi, result ; esi points to buffer for result
loop2:
cmp byte ptr [esi], '0'
jne done2
inc esi ; Skip leading zero
dec ecx ; Decrement digit count
jmp loop2
done2:
; Restore registers and stack frame
pop ebx
pop esi
pop ebp
ret
intToStr ENDP
; Writes a null-terminated string to standard output
; Assumes eax points to the string
WriteString PROC
; Initialize stack frame
push ebp
mov ebp, esp
; Write string to standard output
push eax ; Push string address on stack
call [printf]
; Clean up stack and return
add esp, 4 ; Pop string address from stack
pop ebp
ret
WriteString ENDP
; Reads an integer from standard input
; Returns the integer in eax
ReadInt PROC
; Initialize stack frame
push ebp
mov ebp, esp
; Allocate buffer for input
push MAX_DIGITS ; Push buffer size on stack
call malloc ; Allocate buffer
add esp, 4 ; Pop buffer size from stack
mov esi, eax ; esi points to buffer
; Read input from standard input
push esi ; Push buffer address on stack
push nPrompt ; Push prompt address on stack
call [scanf]
add esp, 8 ; Pop prompt and buffer addresses from stack
; Convert input to integer
mov ebx, 10 ; Base 10
mov eax, 0 ; Start with zero
loop:
movzx ecx, byte ptr [esi] ; Load digit
cmp ecx, '0'
jl done ; End of input
cmp ecx, '9'
jg done ; End of input
sub ecx, '0' ; Convert to integer
imul eax, ebx ; Multiply by base
add eax, ecx ; Add digit to result
inc esi ; Move to next digit
jmp loop
done:
; Free buffer and return
push esi ; Push buffer address on stack
call free ; Free buffer
add esp, 4 ; Pop buffer address from stack
pop ebp
ret
ReadInt ENDP
; C library functions
extern printf : near
extern scanf : near
extern malloc : near
extern free : near
END main
```
该程序首先提示用户输入一个值大于等于50的整数n,然后计算n!并将结果转换为十进制,最后输出结果。
程序使用`factorial`过程递归地计算n!。当n小于或等于1时,递归停止,返回1。否则,该过程递归调用自身来计算(n-1)!,然后将结果乘以n。在每次递归调用之前,该过程将n保存在堆栈中,以便在递归调用完成后恢复n的值。
在计算n!时,该程序使用一个字符缓冲区来存储结果。计算完成后,该程序将结果转换为一个字符串,然后输出该字符串。
注意,该程序使用了C库函数`malloc`,`free`,`printf`和`scanf`来处理内存分配和输入/输出。这些函数通过使用EXTERN指令在程序开头声明。
相关推荐
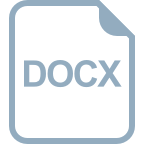














