对你刚才的代码添加注释
时间: 2023-05-30 07:05:24 浏览: 142
# 定义一个函数,函数名为find_median
def find_median(nums1, nums2):
# 将两个列表合并为一个新的列表
nums = nums1 + nums2
# 对新的列表进行排序
nums.sort()
# 获取新的列表的长度
n = len(nums)
# 判断列表长度为奇数还是偶数
if n % 2 == 0:
# 如果列表长度为偶数,取中间两个数的平均值作为中位数
return (nums[n//2-1] + nums[n//2]) / 2
else:
# 如果列表长度为奇数,取中间的数作为中位数
return nums[n//2]
相关问题
如何进行你刚才所说的裁剪,请以代码加注释方式回复
好的,以下是使用Java语言实现图片裁剪的示例代码,注释中详细解释了每个步骤的作用:
```java
import java.awt.Image;
import java.awt.Rectangle;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public class ImageCropper {
public static void main(String[] args) {
try {
// 读取原始图片文件
File input = new File("input.jpg");
BufferedImage originalImage = ImageIO.read(input);
// 定义裁剪区域的坐标和宽高
int x = 50;
int y = 50;
int width = 200;
int height = 200;
// 裁剪图片
BufferedImage croppedImage = originalImage.getSubimage(x, y, width, height);
// 保存裁剪后的图片
File output = new File("output.jpg");
ImageIO.write(croppedImage, "jpg", output);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上述代码中,首先读取了原始图片文件,然后定义了裁剪区域的坐标和宽高,接着使用`getSubimage`方法裁剪了图片,并将裁剪后的图片保存到了输出文件中。
如何进行你刚才所说的裁剪,请以代码加注释方式回复 使用前端vue
好的,以下是使用Vue.js实现图片裁剪的示例代码,注释中详细解释了每个步骤的作用:
```vue
<template>
<div>
<!-- 原始图片 -->
<img ref="originalImage" :src="imageUrl" alt="Original Image" />
<!-- 裁剪区域 -->
<div ref="croppingArea" v-bind:style="{ width: cropWidth + 'px', height: cropHeight + 'px', top: cropTop + 'px', left: cropLeft + 'px' }">
<div class="cropping-area-overlay"></div>
<div class="cropping-area-handle top-left"></div>
<div class="cropping-area-handle top-right"></div>
<div class="cropping-area-handle bottom-left"></div>
<div class="cropping-area-handle bottom-right"></div>
</div>
<!-- 裁剪后的图片 -->
<img ref="croppedImage" :src="croppedImageUrl" alt="Cropped Image" />
</div>
</template>
<script>
export default {
data() {
return {
imageUrl: 'input.jpg',
croppedImageUrl: '',
cropWidth: 200,
cropHeight: 200,
cropTop: 50,
cropLeft: 50,
isDragging: false,
dragStartX: 0,
dragStartY: 0,
dragCropLeft: 0,
dragCropTop: 0
}
},
mounted() {
// 监听裁剪区域的拖拽事件
this.$refs.croppingArea.addEventListener('mousedown', this.handleMouseDown)
this.$refs.croppingArea.addEventListener('mouseup', this.handleMouseUp)
this.$refs.croppingArea.addEventListener('mousemove', this.handleMouseMove)
},
methods: {
handleMouseDown(e) {
// 获取裁剪区域的位置和大小
const croppingArea = this.$refs.croppingArea.getBoundingClientRect()
const { left, top } = croppingArea
const { width, height } = croppingArea
// 计算鼠标在裁剪区域中的相对位置
const x = e.clientX - left
const y = e.clientY - top
// 判断鼠标是否在裁剪区域的边框上
const isTopLeft = x < 10 && y < 10
const isTopRight = x > width - 10 && y < 10
const isBottomLeft = x < 10 && y > height - 10
const isBottomRight = x > width - 10 && y > height - 10
// 如果是,则开始拖拽
if (isTopLeft || isTopRight || isBottomLeft || isBottomRight) {
this.isDragging = true
this.dragStartX = e.clientX
this.dragStartY = e.clientY
this.dragCropLeft = this.cropLeft
this.dragCropTop = this.cropTop
}
},
handleMouseUp() {
// 停止拖拽
this.isDragging = false
},
handleMouseMove(e) {
if (this.isDragging) {
// 计算鼠标拖拽的距离
const deltaX = e.clientX - this.dragStartX
const deltaY = e.clientY - this.dragStartY
// 更新裁剪区域的位置
if (this.dragCropLeft + deltaX >= 0 && this.dragCropLeft + deltaX + this.cropWidth <= this.$refs.originalImage.width) {
this.cropLeft = this.dragCropLeft + deltaX
}
if (this.dragCropTop + deltaY >= 0 && this.dragCropTop + deltaY + this.cropHeight <= this.$refs.originalImage.height) {
this.cropTop = this.dragCropTop + deltaY
}
}
},
cropImage() {
// 创建一个Canvas对象
const canvas = document.createElement('canvas')
const ctx = canvas.getContext('2d')
// 设置Canvas对象的大小
canvas.width = this.cropWidth
canvas.height = this.cropHeight
// 绘制被裁剪的图片到Canvas对象上
ctx.drawImage(this.$refs.originalImage, this.cropLeft, this.cropTop, this.cropWidth, this.cropHeight, 0, 0, this.cropWidth, this.cropHeight)
// 获取裁剪后的图片的Base64编码
const croppedImageUrl = canvas.toDataURL('image/jpeg')
// 更新裁剪后的图片的URL
this.croppedImageUrl = croppedImageUrl
}
}
}
</script>
<style>
.cropping-area-overlay {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
}
.cropping-area-handle {
position: absolute;
z-index: 1;
width: 20px;
height: 20px;
background-color: white;
border: 1px solid black;
}
.cropping-area-handle.top-left {
top: -10px;
left: -10px;
cursor: nwse-resize;
}
.cropping-area-handle.top-right {
top: -10px;
right: -10px;
cursor: nesw-resize;
}
.cropping-area-handle.bottom-left {
bottom: -10px;
left: -10px;
cursor: nesw-resize;
}
.cropping-area-handle.bottom-right {
bottom: -10px;
right: -10px;
cursor: nwse-resize;
}
</style>
```
在上述代码中,首先渲染了原始图片和裁剪区域,然后监听了裁剪区域的鼠标拖拽事件,在拖拽时更新了裁剪区域的位置和大小,并使用Canvas对象裁剪了原始图片。最后,将裁剪后的图片以Base64编码的形式显示在页面上。
阅读全文
相关推荐
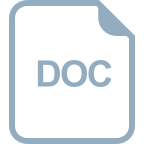
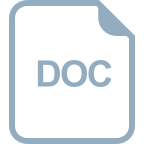
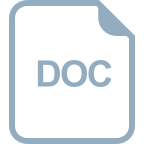
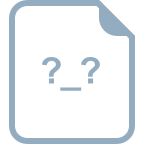





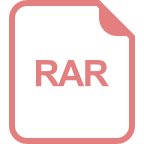
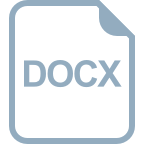
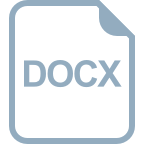
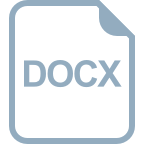



