用Python写一个脚本,识别Verilog中的include文件,include文件跟Verilog文件不在同一个文件夹
时间: 2024-02-17 07:00:45 浏览: 71
好的,我可以为您编写一个Python脚本,用于识别Verilog中的include文件。以下是实现的思路:
1. 读取Verilog文件,查找所有的include语句。
2. 对于每个include语句,解析文件路径,确定include文件的绝对路径。
3. 检查include文件是否存在,如果存在,则将其路径添加到列表中。
4. 对于每个include文件,递归调用上述步骤,直到所有include文件被处理。
下面是基本实现:
```python
import os
def find_includes(verilog_file, include_paths=None):
"""
查找Verilog文件中的include文件,并返回所有include文件的绝对路径
:param verilog_file: Verilog文件路径
:param include_paths: include文件搜索路径列表,默认为None
:return: 包含所有include文件路径的列表
"""
# 如果未提供include搜索路径,则使用当前目录
if include_paths is None:
include_paths = [os.getcwd()]
# 读取Verilog文件内容
with open(verilog_file, 'r') as f:
content = f.read()
# 查找所有include语句
includes = []
for line in content.split('\n'):
if line.startswith('`include'):
# 解析include语句中的文件路径
include_file = line.split()[1].strip('"')
# 确定include文件的绝对路径
for path in include_paths:
abs_path = os.path.abspath(os.path.join(path, include_file))
if os.path.exists(abs_path):
includes.append(abs_path)
break
# 处理include文件中的include语句
if abs_path:
includes += find_includes(abs_path, include_paths)
return includes
```
使用示例:
```python
verilog_file = './test.v'
include_paths = ['./includes', '../common/includes']
includes = find_includes(verilog_file, include_paths)
print(includes)
```
在此示例中,我们搜索名为`test.v`的Verilog文件,并在`./includes`和`../common/includes`目录中搜索include文件。脚本将返回包含所有include文件路径的列表。
阅读全文
相关推荐
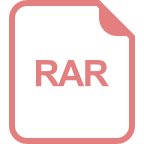





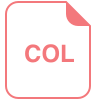





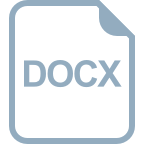