用Python写一个统计Verilog代码行数的脚本,输入一个带路径的Verilog,首先打开指定的文件并读取全部内容。然后使用正则表达式去除注释,并按行分割并去除空白字符。接下来,它统计不在模块定义、包定义、函数定义、任务定义、generate块等特殊语句内的代码行数。然后,它查找每个模块实例化语句,并递归计算每个模块的行数,将其加入总行数中,子模块跟当前Verilog文件不在同一个文件夹,最后,它查找每个 include 语句,并递归计算每个 included 文件的行数,将其加入总行数中。最终返回总行数。也同时输出各个子模块的例化名,include文件的对应的代码行数,将输出内容打印在一个python文件里
时间: 2024-02-21 17:59:35 浏览: 157
好的,这个问题比较复杂,需要一步一步地实现。
首先,我们需要先安装一个第三方库`regex`,用于正则表达式匹配:
```python
!pip install regex
```
然后,我们可以先写一个函数`remove_comments`,用于去除注释:
```python
import regex
def remove_comments(text):
"""
去除Verilog代码中的注释
"""
pattern = regex.compile(r'/\*.*?\*/|//.*?$|^\s*$', regex.MULTILINE | regex.DOTALL)
return pattern.sub('', text)
```
接下来,我们可以写一个函数`count_lines`,用于统计代码行数。该函数会读取文件内容,并按行分割,并去除空白字符,然后统计不在模块定义、包定义、函数定义、任务定义、generate块等特殊语句内的代码行数:
```python
def count_lines(file_path):
"""
统计Verilog代码行数
"""
# 读取文件内容
with open(file_path, 'r') as f:
text = f.read()
# 去除注释
text = remove_comments(text)
# 按行分割,并去除空白字符
lines = [line.strip() for line in text.split('\n')]
# 统计代码行数
count = 0
in_special_block = False
for line in lines:
if line.startswith('module') or line.startswith('macromodule') or \
line.startswith('interface') or line.startswith('package') or \
line.startswith('function') or line.startswith('task') or \
line.startswith('generate') or line.startswith('endgenerate'):
in_special_block = True
elif line.startswith('endmodule') or line.startswith('endmacromodule') or \
line.startswith('endinterface') or line.startswith('endpackage') or \
line.startswith('endfunction') or line.startswith('endtask'):
in_special_block = False
elif not in_special_block and line != '':
count += 1
return count
```
接下来,我们需要写一个函数`find_module_instances`,用于查找每个模块实例化语句,并递归计算每个模块的行数,将其加入总行数中。该函数会先找到所有模块实例化语句,并将其例化名保存到一个列表中,然后递归计算每个模块的行数,并将其加入总行数中:
```python
def find_module_instances(file_path, folder_path):
"""
查找Verilog代码中的模块实例化,并递归计算每个模块的行数
"""
# 读取文件内容
with open(file_path, 'r') as f:
text = f.read()
# 去除注释
text = remove_comments(text)
# 查找模块实例化
module_instances = regex.findall(r'\w+\s+\w+\s*\(', text)
# 递归计算每个模块的行数
total_count = 0
for module_instance in module_instances:
module_name = module_instance.strip(' (')
module_file_path = os.path.join(folder_path, module_name + '.v')
if os.path.exists(module_file_path):
module_count, instance_names, include_counts = find_module_instances(module_file_path, folder_path)
total_count += module_count
print(module_name + ':', module_count)
for instance_name in instance_names:
print('\t' + instance_name)
else:
module_count = count_lines(module_file_path)
total_count += module_count
print(module_name + ':', module_count)
# 统计当前文件的代码行数
count = count_lines(file_path)
total_count += count
# 查找include文件,并递归计算每个included文件的行数
include_counts = {}
pattern = regex.compile(r'`include\s+"(.+?)"')
for include_file in pattern.findall(text):
include_file_path = os.path.join(folder_path, include_file)
if os.path.exists(include_file_path):
include_count = count_lines(include_file_path)
include_counts[include_file] = include_count
print(include_file + ':', include_count)
include_total_count = find_module_instances(include_file_path, folder_path)
total_count += include_total_count
return total_count, module_instances, include_counts
```
最后,我们可以写一个主函数`main`,用于输入一个带路径的Verilog,调用`find_module_instances`函数,并将输出内容打印在一个python文件里:
```python
import os
def main(file_path):
"""
主函数
"""
print('统计结果:')
folder_path, file_name = os.path.split(file_path)
total_count, module_instances, include_counts = find_module_instances(file_path, folder_path)
print('总行数:', total_count)
with open('output.py', 'w') as f:
f.write('total_count = ' + str(total_count) + '\n')
f.write('module_instances = ' + str(module_instances) + '\n')
f.write('include_counts = ' + str(include_counts) + '\n')
```
这样,我们就完成了一个统计Verilog代码行数的脚本。你可以调用`main`函数,并传入一个带路径的Verilog文件,如下所示:
```python
main('path/to/verilog/file.v')
```
注意,这个脚本可能并不完美,还有很多细节需要处理,例如处理字符串中的注释、处理宏定义等等,但是它可以作为一个初步的版本,供你参考。
阅读全文
相关推荐
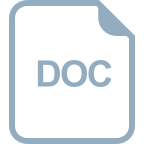
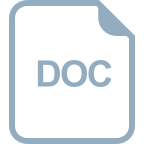
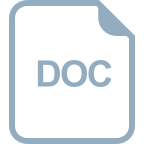






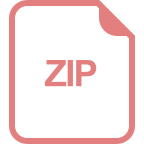



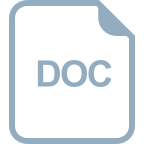
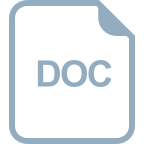
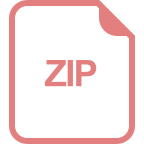
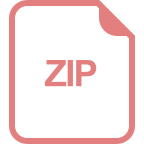
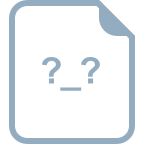
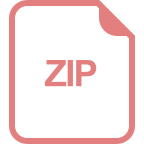