用Python代码设计一个字符界面人机对战的五子棋游戏
时间: 2023-06-16 17:08:00 浏览: 121
好的,以下是一个简单的五子棋游戏的代码,采用Python的`curses`库实现了字符界面的交互:
```python
import curses
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋子的字符表示
BLACK = "●"
WHITE = "○"
# 定义棋盘的边框和网格线的字符表示
BORDER_CHAR = "+"
HLINE_CHAR = "-"
VLINE_CHAR = "|"
# 定义棋子颜色
BLACK_COLOR = curses.COLOR_WHITE
WHITE_COLOR = curses.COLOR_BLACK
# 初始化curses
screen = curses.initscr()
curses.start_color()
curses.use_default_colors()
curses.noecho()
curses.cbreak()
screen.keypad(True)
# 定义颜色对
curses.init_pair(1, BLACK_COLOR, curses.COLOR_GREEN)
curses.init_pair(2, WHITE_COLOR, curses.COLOR_GREEN)
# 定义棋盘数组
board = [[0 for i in range(BOARD_SIZE)] for j in range(BOARD_SIZE)]
# 定义当前棋手
current_player = BLACK
# 定义游戏是否结束的标志
game_over = False
# 定义检查是否胜利的函数
def check_win(player):
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == player:
# 检查横向是否胜利
if j + 4 < BOARD_SIZE and board[i][j + 1] == player and board[i][j + 2] == player and board[i][j + 3] == player and board[i][j + 4] == player:
return True
# 检查纵向是否胜利
if i + 4 < BOARD_SIZE and board[i + 1][j] == player and board[i + 2][j] == player and board[i + 3][j] == player and board[i + 4][j] == player:
return True
# 检查右上到左下是否胜利
if i + 4 < BOARD_SIZE and j + 4 < BOARD_SIZE and board[i + 1][j + 1] == player and board[i + 2][j + 2] == player and board[i + 3][j + 3] == player and board[i + 4][j + 4] == player:
return True
# 检查左上到右下是否胜利
if i - 4 >= 0 and j + 4 < BOARD_SIZE and board[i - 1][j + 1] == player and board[i - 2][j + 2] == player and board[i - 3][j + 3] == player and board[i - 4][j + 4] == player:
return True
return False
# 定义绘制棋盘的函数
def draw_board():
screen.clear()
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
# 绘制边框
if i == 0 and j == 0:
screen.addstr(i, j, BORDER_CHAR)
elif i == 0 and j == BOARD_SIZE - 1:
screen.addstr(i, j, BORDER_CHAR)
elif i == BOARD_SIZE - 1 and j == 0:
screen.addstr(i, j, BORDER_CHAR)
elif i == BOARD_SIZE - 1 and j == BOARD_SIZE - 1:
screen.addstr(i, j, BORDER_CHAR)
elif i == 0 or i == BOARD_SIZE - 1:
screen.addstr(i, j, HLINE_CHAR)
elif j == 0 or j == BOARD_SIZE - 1:
screen.addstr(i, j, VLINE_CHAR)
else:
# 绘制棋子
if board[i][j] == BLACK:
screen.addstr(i, j, BLACK, curses.color_pair(1))
elif board[i][j] == WHITE:
screen.addstr(i, j, WHITE, curses.color_pair(2))
# 定义主循环
while not game_over:
# 绘制棋盘
draw_board()
# 获取用户输入
key = screen.getch()
if key == curses.KEY_UP:
# 向上移动光标
cursor_y -= 1
if cursor_y < 0:
cursor_y = 0
elif key == curses.KEY_DOWN:
# 向下移动光标
cursor_y += 1
if cursor_y >= BOARD_SIZE:
cursor_y = BOARD_SIZE - 1
elif key == curses.KEY_LEFT:
# 向左移动光标
cursor_x -= 1
if cursor_x < 0:
cursor_x = 0
elif key == curses.KEY_RIGHT:
# 向右移动光标
cursor_x += 1
if cursor_x >= BOARD_SIZE:
cursor_x = BOARD_SIZE - 1
elif key == ord(" "):
# 如果当前位置没有棋子,则落子
if board[cursor_y][cursor_x] == 0:
board[cursor_y][cursor_x] = current_player
# 检查是否胜利
if check_win(current_player):
game_over = True
else:
# 切换棋手
if current_player == BLACK:
current_player = WHITE
else:
current_player = BLACK
# 显示胜利者
draw_board()
if current_player == BLACK:
screen.addstr(BOARD_SIZE + 1, 0, "BLACK WINS!")
else:
screen.addstr(BOARD_SIZE + 1, 0, "WHITE WINS!")
# 等待用户输入
screen.getch()
# 关闭curses
curses.nocbreak()
screen.keypad(False)
curses.echo()
curses.endwin()
```
这个游戏的基本逻辑是:使用光标键移动光标,按空格键落子,检查是否胜利,然后切换棋手,直到有一方胜利为止。注意,这个代码需要在终端中运行,否则可能无法正常工作。
阅读全文
相关推荐
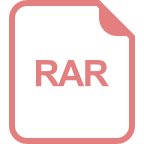


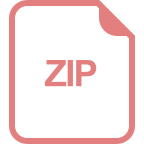
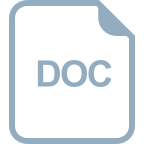
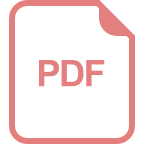
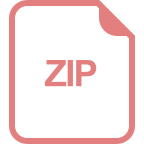
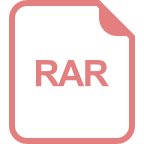
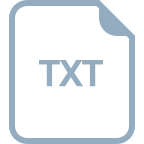
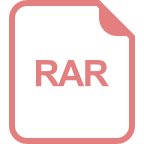
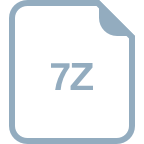
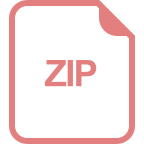
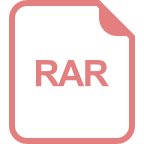
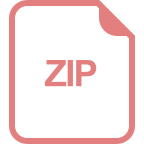
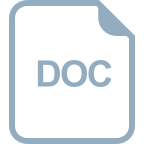
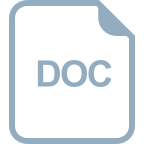
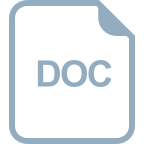