'datetime.date' object has no attribute 'timedelta'
时间: 2023-09-13 08:04:49 浏览: 330
This error occurs when you try to use the timedelta method on a datetime.date object. The timedelta method is only available for datetime.datetime objects.
To fix this error, you can convert the datetime.date object to a datetime.datetime object using the datetime.combine() method. Here's an example:
```
import datetime
date_obj = datetime.date(2021, 10, 1)
datetime_obj = datetime.datetime.combine(date_obj, datetime.datetime.min.time())
# now you can use the timedelta method
delta = datetime.timedelta(days=1)
new_date = datetime_obj + delta
print(new_date.date())
```
This will output "2021-10-02", which is the next day after the original date object.
相关问题
'datetime.date' object has no attribute 'timedelta
The error message "datetime.date' object has no attribute 'timedelta" indicates that you are trying to use the 'timedelta' method on an object of the 'date' class in Python's 'datetime' module. However, the 'date' class does not have a 'timedelta' method.
To use the 'timedelta' method, you need to create an object of the 'datetime' class instead of the 'date' class. The 'datetime' class has both 'date' and 'time' attributes, and you can perform arithmetic operations like addition and subtraction on these attributes using the 'timedelta' method.
Here is an example of how to create a 'datetime' object and use the 'timedelta' method:
```
import datetime
# create a datetime object
dt = datetime.datetime(2021, 9, 1, 10, 30, 0)
# add one day to the datetime object
dt_plus_one_day = dt + datetime.timedelta(days=1)
# subtract two hours from the datetime object
dt_minus_two_hours = dt - datetime.timedelta(hours=2)
```
In this example, we created a 'datetime' object representing September 1st, 2021 at 10:30 AM. We then added one day to this object using the 'timedelta' method with the 'days' argument. Finally, we subtracted two hours from the object using the 'timedelta' method with the 'hours' argument.
AttributeError: type object 'datetime.datetime' has no attribute 'timedelta'
这个错误是因为你尝试在 `datetime.datetime` 上调用 `timedelta` 方法,但是 `timedelta` 是 `datetime` 模块中另一个类的方法。
你可以通过以下方式使用 `timedelta`:
```python
import datetime
# 创建一个时间差
delta = datetime.timedelta(days=1)
# 将时间差加到日期上
date = datetime.datetime.now() + delta
```
如果你仍然遇到问题,请检查你的 Python 版本,因为在某些旧版本中可能存在此问题。
阅读全文
相关推荐
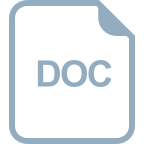
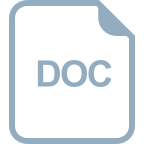
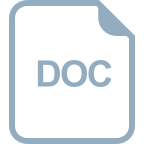













