用python写个飞机大战代码
时间: 2023-12-18 15:03:53 浏览: 80
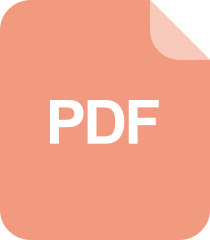
500行代码使用python写个微信小游戏飞机大战游戏
好的,以下是一个简单的飞机大战游戏代码:
```python
import pygame
import random
# 初始化
pygame.init()
# 游戏窗口大小
WIDTH 480
HEIGHT = 600
# 创建游戏窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
# 标题和图标
pygame.display.set_caption("飞机大战")
icon = pygame.image.load("icon.png")
pygame.display.set_icon(icon)
# 背景
background = pygame.image.load("background.png")
# 飞机
player_img = pygame.image.load("player.png")
player_x = WIDTH // 2 - 32
player_y = HEIGHT - 96
player_speed = 5
# 子弹
bullet_img = pygame.image.load("bullet.png")
bullet_speed = 10
bullet_list = []
# 敌机
enemy_img = pygame.image.load("enemy.png")
enemy_list = []
enemy_speed = 5
enemy_spawn_rate = 50
# 飞机函数
def draw_player(x, y):
screen.blit(player_img, (x, y))
# 子弹函数
def draw_bullet(x, y):
screen.blit(bullet_img, (x, y))
# 敌机函数
def draw_enemy(x, y):
screen.blit(enemy_img, (x, y))
# 检测碰撞
def is_collision(x1, y1, x2, y2):
distance = ((x1 - x2) ** 2 + (y1 - y2) ** 2) ** 0.5
if distance < 64:
return True
else:
return False
# 游戏循环
running = True
while running:
# 背景色
screen.fill((0, 0, 0))
# 背景图片
screen.blit(background, (0, 0))
# 事件循环
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 键盘操作
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
player_x -= player_speed
if event.key == pygame.K_RIGHT:
player_x += player_speed
if event.key == pygame.K_SPACE:
bullet_list.append([player_x + 32, player_y - 32])
# 移动飞机
if player_x < 0:
player_x = 0
elif player_x > WIDTH - 64:
player_x = WIDTH - 64
# 移动子弹
for bullet in bullet_list:
bullet[1] -= bullet_speed
draw_bullet(bullet[0], bullet[1])
# 生成敌机
if random.randint(0, 100) < enemy_spawn_rate:
enemy_list.append([random.randint(0, WIDTH - 64), 0])
# 移动敌机
for enemy in enemy_list:
enemy[1] += enemy_speed
draw_enemy(enemy[0], enemy[1])
# 检测碰撞
if is_collision(enemy[0], enemy[1], player_x, player_y):
running = False
# 检测子弹击中敌机
for bullet in bullet_list:
if is_collision(enemy[0], enemy[1], bullet[0], bullet[1]):
bullet_list.remove(bullet)
enemy_list.remove(enemy)
# 绘制飞机
draw_player(player_x, player_y)
# 更新窗口
pygame.display.update()
# 退出游戏
pygame.quit()
```
注意:代码中的图片和图标需要自己准备,并且放在与代码文件相同的目录下。
阅读全文
相关推荐
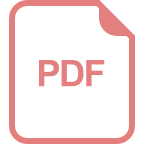










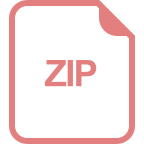
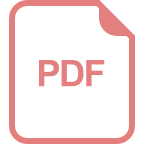
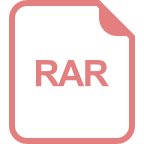
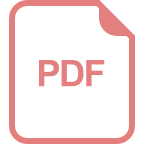
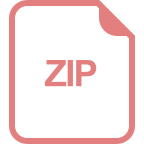