手机版c语言编译器简单发出声音的代码
时间: 2023-09-14 17:07:46 浏览: 60
以下是一个简单的C语言程序,用于在手机上发出一段音频:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/soundcard.h>
#define BUF_SIZE 4096
int main(int argc, char **argv) {
int fd, arg, status;
char *buf;
double freq = 440.0; // frequency of the tone
int duration = 1; // duration of the tone in seconds
// open the sound device
fd = open("/dev/dsp", O_WRONLY);
if (fd < 0) {
perror("open");
exit(1);
}
// set the format of the sound data
arg = 0;
arg |= AFMT_S16_LE; // 16-bit signed little-endian
arg |= SNDCTL_DSP_SETFMT;
status = ioctl(fd, SNDCTL_DSP_SETFMT, &arg);
if (status < 0) {
perror("ioctl");
exit(1);
}
// set the number of channels
arg = 1; // mono
arg |= SNDCTL_DSP_CHANNELS;
status = ioctl(fd, SNDCTL_DSP_CHANNELS, &arg);
if (status < 0) {
perror("ioctl");
exit(1);
}
// set the sampling rate
arg = 44100; // 44.1 kHz
arg |= SNDCTL_DSP_SPEED;
status = ioctl(fd, SNDCTL_DSP_SPEED, &arg);
if (status < 0) {
perror("ioctl");
exit(1);
}
// allocate the buffer for the sound data
buf = malloc(BUF_SIZE);
if (buf == NULL) {
perror("malloc");
exit(1);
}
// generate the sound data
int samples = arg * duration;
double factor = 2.0 * M_PI * freq / arg;
int i, j;
for (i = 0; i < samples; i += BUF_SIZE / 2) {
for (j = 0; j < BUF_SIZE / 2 && i + j < samples; j++) {
short value = (short) (32767.0 * sin(i * factor));
buf[2 * j] = value & 0xff;
buf[2 * j + 1] = (value >> 8) & 0xff;
}
write(fd, buf, j * 2);
usleep(1000);
}
// free the buffer and close the sound device
free(buf);
close(fd);
return 0;
}
```
该程序使用Linux的音频设备(`/dev/dsp`)生成一个简单的正弦波,并将其写入设备以发出声音。该程序使用了如下的函数和库:
- `open`:打开设备文件
- `ioctl`:设置设备参数
- `malloc`:分配内存
- `sin`:计算正弦函数
- `write`:向设备写入数据
- `usleep`:暂停程序的执行一段时间
在这个程序中,我们首先打开音频设备(`/dev/dsp`)。然后,我们设置音频数据的格式、通道数和采样率。在这个例子中,我们使用了16位有符号的小端格式,单声道和44.1kHz的采样率。接下来,我们使用malloc函数分配一个缓冲区,用于存储音频数据。然后,我们生成正弦波数据,并将其写入设备以发出声音。最后,我们释放缓冲区,并关闭设备。
注意,这个程序只是一个简单的例子,它只能发出一个固定频率的正弦波。如果你想要更多的控制,比如生成一个具有不同频率、振幅和持续时间的波形,你需要编写更复杂的代码。
相关推荐
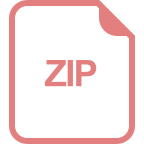















