编写一个基于stm32的四路抢答器的程序
时间: 2023-07-25 16:03:40 浏览: 106
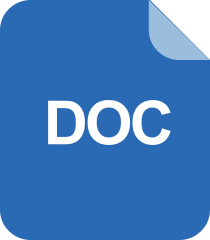
基于STM32的四路抢答器课程设计

由于篇幅限制,我无法在这里提供完整的代码,但可以提供一个基本的程序框架,供您参考:
```
#include "stm32f10x.h"
// 定义抢答器按键对应的GPIO口和引脚号
#define KEY1_GPIO_CLK RCC_APB2Periph_GPIOA
#define KEY1_GPIO_PORT GPIOA
#define KEY1_GPIO_PIN GPIO_Pin_0
#define KEY2_GPIO_CLK RCC_APB2Periph_GPIOA
#define KEY2_GPIO_PORT GPIOA
#define KEY2_GPIO_PIN GPIO_Pin_1
#define KEY3_GPIO_CLK RCC_APB2Periph_GPIOA
#define KEY3_GPIO_PORT GPIOA
#define KEY3_GPIO_PIN GPIO_Pin_2
#define KEY4_GPIO_CLK RCC_APB2Periph_GPIOA
#define KEY4_GPIO_PORT GPIOA
#define KEY4_GPIO_PIN GPIO_Pin_3
// 定义显示屏对应的GPIO口和引脚号
#define LCD_GPIO_CLK RCC_APB2Periph_GPIOB
#define LCD_GPIO_PORT GPIOB
#define LCD_RS_GPIO_PIN GPIO_Pin_5
#define LCD_RW_GPIO_PIN GPIO_Pin_6
#define LCD_E_GPIO_PIN GPIO_Pin_7
#define LCD_DB0_GPIO_PIN GPIO_Pin_8
#define LCD_DB1_GPIO_PIN GPIO_Pin_9
#define LCD_DB2_GPIO_PIN GPIO_Pin_10
#define LCD_DB3_GPIO_PIN GPIO_Pin_11
#define LCD_DB4_GPIO_PIN GPIO_Pin_12
#define LCD_DB5_GPIO_PIN GPIO_Pin_13
#define LCD_DB6_GPIO_PIN GPIO_Pin_14
#define LCD_DB7_GPIO_PIN GPIO_Pin_15
// 定义蜂鸣器对应的GPIO口和引脚号
#define BEEP_GPIO_CLK RCC_APB2Periph_GPIOB
#define BEEP_GPIO_PORT GPIOB
#define BEEP_GPIO_PIN GPIO_Pin_0
// 定义抢答器个数
#define NUM_OF_QUES 4
// 定义抢答状态
typedef enum {
WAIT_FOR_KEY, // 等待按键
KEY_PRESSED, // 按键已按下
KEY_RELEASED, // 按键已松开
ANSWERED // 已回答
} AnswerState;
// 定义抢答器结构体
typedef struct {
GPIO_TypeDef* GPIO_Port; // GPIO口
uint16_t GPIO_Pin; // 引脚号
uint8_t ID; // 抢答器编号
AnswerState State; // 抢答状态
uint32_t PressTime; // 按键按下时间
} Question;
// 定义抢答器数组
Question ques[NUM_OF_QUES];
// 初始化抢答器
void InitQuestions(void)
{
RCC_APB2PeriphClockCmd(KEY1_GPIO_CLK | KEY2_GPIO_CLK | KEY3_GPIO_CLK | KEY4_GPIO_CLK, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
// 初始化按键GPIO口
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Pin = KEY1_GPIO_PIN;
GPIO_Init(KEY1_GPIO_PORT, &GPIO_InitStructure);
ques[0].GPIO_Port = KEY1_GPIO_PORT;
ques[0].GPIO_Pin = KEY1_GPIO_PIN;
ques[0].ID = 1;
ques[0].State = WAIT_FOR_KEY;
GPIO_InitStructure.GPIO_Pin = KEY2_GPIO_PIN;
GPIO_Init(KEY2_GPIO_PORT, &GPIO_InitStructure);
ques[1].GPIO_Port = KEY2_GPIO_PORT;
ques[1].GPIO_Pin = KEY2_GPIO_PIN;
ques[1].ID = 2;
ques[1].State = WAIT_FOR_KEY;
GPIO_InitStructure.GPIO_Pin = KEY3_GPIO_PIN;
GPIO_Init(KEY3_GPIO_PORT, &GPIO_InitStructure);
ques[2].GPIO_Port = KEY3_GPIO_PORT;
ques[2].GPIO_Pin = KEY3_GPIO_PIN;
ques[2].ID = 3;
ques[2].State = WAIT_FOR_KEY;
GPIO_InitStructure.GPIO_Pin = KEY4_GPIO_PIN;
GPIO_Init(KEY4_GPIO_PORT, &GPIO_InitStructure);
ques[3].GPIO_Port = KEY4_GPIO_PORT;
ques[3].GPIO_Pin = KEY4_GPIO_PIN;
ques[3].ID = 4;
ques[3].State = WAIT_FOR_KEY;
}
// 初始化显示屏
void InitLCD(void)
{
RCC_APB2PeriphClockCmd(LCD_GPIO_CLK, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
// 初始化显示屏GPIO口
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Pin = LCD_RS_GPIO_PIN | LCD_RW_GPIO_PIN | LCD_E_GPIO_PIN;
GPIO_Init(LCD_GPIO_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = LCD_DB0_GPIO_PIN | LCD_DB1_GPIO_PIN | LCD_DB2_GPIO_PIN | LCD_DB3_GPIO_PIN | LCD_DB4_GPIO_PIN | LCD_DB5_GPIO_PIN | LCD_DB6_GPIO_PIN | LCD_DB7_GPIO_PIN;
GPIO_Init(LCD_GPIO_PORT, &GPIO_InitStructure);
// 显示屏初始化代码,具体实现可以参考LCD1602的初始化代码
}
// 初始化蜂鸣器
void InitBEEP(void)
{
RCC_APB2PeriphClockCmd(BEEP_GPIO_CLK, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
// 初始化蜂鸣器GPIO口
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Pin = BEEP_GPIO_PIN;
GPIO_Init(BEEP_GPIO_PORT, &GPIO_InitStructure);
}
// 检测按键状态
AnswerState CheckKeyState(Question* q)
{
if (GPIO_ReadInputDataBit(q->GPIO_Port, q->GPIO_Pin) == Bit_RESET) {
if (q->State == WAIT_FOR_KEY) {
q->State = KEY_PRESSED;
q->PressTime = SysTick->VAL;
}
} else {
if (q->State == KEY_PRESSED) {
q->State = KEY_RELEASED;
}
}
return q->State;
}
// 检测抢答状态
void CheckAnswerState(void)
{
uint8_t i;
for (i = 0; i < NUM_OF_QUES; i++) {
Question* q = &ques[i];
switch (q->State) {
case WAIT_FOR_KEY:
break;
case KEY_PRESSED:
if ((SysTick->VAL - q->PressTime) > 100) { // 检测是否长按
q->State = WAIT_FOR_KEY;
}
break;
case KEY_RELEASED:
q->State = ANSWERED;
// 显示抢答结果
// 发送抢答结果到主控端
break;
case ANSWERED:
break;
}
}
}
int main(void)
{
// 初始化系统时钟
RCC_DeInit();
SystemInit();
// 初始化抢答器、显示屏、蜂鸣器
InitQuestions();
InitLCD();
InitBEEP();
// 系统滴答定时器初始化
if (SysTick_Config(SystemCoreClock / 1000)) {
while (1);
}
while (1) {
// 检测按键状态
CheckAnswerState();
// 显示抢答器状态
// 显示倒计时时间
}
}
```
需要注意的是,以上仅是一个基本的程序框架,具体的实现还需要根据实际需求进行修改和完善。同时,还需要添加相应的中断处理函数和串口通信代码等。
阅读全文
相关推荐
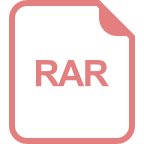
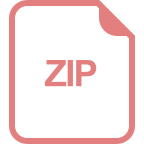















