vhdl写一个流水灯,从0点亮到7再从7点亮到0
时间: 2024-03-11 09:46:54 浏览: 10
好的,以下是一个简单的 VHDL 代码,实现一个流水灯,从 0 点亮到 7 再从 7 点亮到 0:
```vhdl
-- 定义一个 8 位的信号,用于表示流水灯的状态
signal leds: std_logic_vector(7 downto 0) := "00000000";
-- 定义一个计数器,用于控制流水灯的方向和速度
signal count: integer range 0 to 15 := 0;
-- 定义一个时钟信号,用于控制流水灯的频率
signal clk: std_logic := '0';
-- 时钟分频模块,将输入时钟分频为一个较低的频率
entity clk_divider is
port(
clk_in: in std_logic;
clk_out: out std_logic
);
end entity clk_divider;
architecture rtl of clk_divider is
signal counter: integer range 0 to 49999999 := 0; -- 2Hz 的时钟
begin
process(clk_in)
begin
if rising_edge(clk_in) then
counter <= counter + 1;
if counter = 49999999 then
counter <= 0;
clk_out <= not clk_out; -- 时钟反转
end if;
end if;
end process;
end architecture rtl;
-- 流水灯模块,控制 8 个 LED 的点亮状态
entity led_driver is
port(
clk: in std_logic;
leds: out std_logic_vector(7 downto 0)
);
end entity led_driver;
architecture rtl of led_driver is
begin
process(clk)
begin
if rising_edge(clk) then
-- 根据计数器的值控制流水灯的方向和速度
case count is
when 0 to 6 =>
leds <= leds(6 downto 0) & '0';
count <= count + 1;
when 7 =>
leds <= "10000000";
count <= 8;
when 8 to 14 =>
leds <= '0' & leds(7 downto 1);
count <= count + 1;
when 15 =>
leds <= "00000001";
count <= 0;
end case;
end if;
end process;
end architecture rtl;
-- 顶层模块,将时钟和 LED 信号连接起来
entity top is
port(
clk_in: in std_logic;
leds: out std_logic_vector(7 downto 0)
);
end entity top;
architecture rtl of top is
signal clk_div: std_logic;
begin
-- 实例化时钟分频模块
clk_div_inst: entity work.clk_divider
port map(
clk_in => clk_in,
clk_out => clk_div
);
-- 实例化流水灯模块
led_driver_inst: entity work.led_driver
port map(
clk => clk_div,
leds => leds
);
end architecture rtl;
```
在这个代码中,我们定义了一个 `leds` 信号,用于表示 8 个 LED 灯的状态。我们还定义了一个计数器 `count`,用于控制流水灯的方向和速度。在时钟分频模块中,我们将输入时钟 `clk_in` 分频为一个 2Hz 的时钟 `clk_out`。在流水灯模块中,我们根据计数器的值控制流水灯的方向和速度。最后,在顶层模块中,我们将时钟和 LED 信号连接起来,并实例化时钟分频模块和流水灯模块。
相关推荐
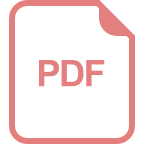










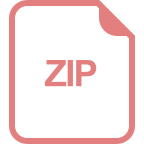