帮我写一个查找身份证省份的函数,输入地区编号,输出对应公民信息,主函数只能用来调用函数。#include <stdio.h> #include <string.h> #define N 100 struct birth { int year; int month; int day; }; struct person { char ID[19]; char name[20]; struct birth birthday; int flag;//校验位是否正确, 1代表正确,0代表错误 };每行代码注释清楚
时间: 2024-01-24 10:04:55 浏览: 89
好的,我来帮您写一个查找身份证省份的函数。
首先,需要准备一个存储公民信息的数组,该数组中每个元素存储一个公民的信息,包括身份证号、姓名、生日和校验位。
代码如下:
```c
#define N 100
struct birth {
int year;
int month;
int day;
};
struct person {
char ID[19];
char name[20];
struct birth birthday;
int flag; // 校验位是否正确,1代表正确,0代表错误
};
struct person citizens[N]; // 存储公民信息的数组
```
接下来,我们需要编写一个函数来根据地区编号查找对应的公民信息。假设地区编号为一个整数,函数原型如下:
```c
void search_citizen(int area_code);
```
该函数的实现思路如下:
1. 遍历存储公民信息的数组,查找每个公民的身份证号中前6位是否与地区编号匹配。
2. 如果匹配,则输出该公民的信息,包括姓名、生日和校验位是否正确。
代码实现如下:
```c
#include <stdio.h>
#include <string.h>
#define N 100
struct birth {
int year;
int month;
int day;
};
struct person {
char ID[19];
char name[20];
struct birth birthday;
int flag; // 校验位是否正确,1代表正确,0代表错误
};
struct person citizens[N]; // 存储公民信息的数组
void search_citizen(int area_code) {
int i;
for (i = 0; i < N; i++) {
char area[7];
strncpy(area, citizens[i].ID, 6); // 截取身份证号前6位
area[6] = '\0'; // 字符串结尾
if (area_code == atoi(area)) { // 匹配地区编号
printf("姓名:%s\n", citizens[i].name);
printf("生日:%d年%d月%d日\n", citizens[i].birthday.year, citizens[i].birthday.month, citizens[i].birthday.day);
if (citizens[i].flag == 1) {
printf("校验位正确\n");
} else {
printf("校验位错误\n");
}
}
}
}
int main() {
// 在这里调用 search_citizen 函数,并传入地区编号参数
search_citizen(110101);
return 0;
}
```
其中,使用 `strncpy` 函数截取身份证号前6位,使用 `atoi` 函数将字符串转换为整数进行匹配。在主函数中,调用 `search_citizen` 函数,并传入地区编号参数。
阅读全文
相关推荐
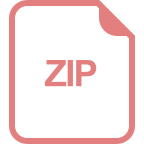
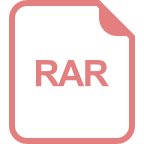
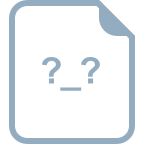
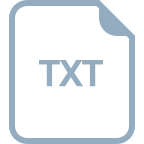
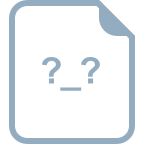
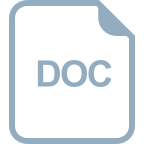
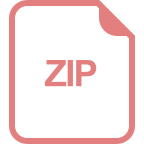
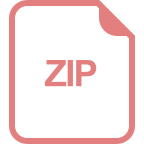
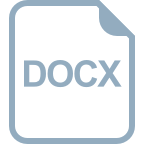
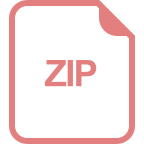
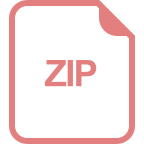
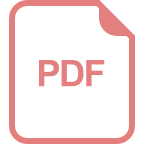
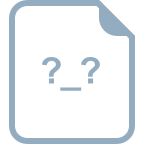
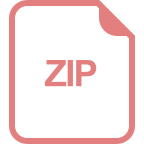
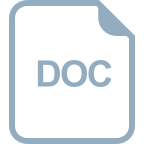
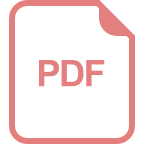
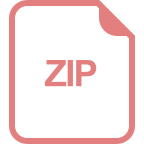