c语言基于离散余弦变换的图像压缩算法
时间: 2023-08-18 17:07:14 浏览: 94
C语言基于离散余弦变换的图像压缩算法通常称为DCT压缩算法。该算法将图像分成小块,然后对每个块进行离散余弦变换(DCT)处理,得到块的频率分量。接着,对这些频率分量进行量化,通过舍弃一些细节信息来压缩数据。最后,通过熵编码将压缩后的数据编码为比特流。在解压缩时,需要将比特流解码为频率分量,然后进行逆量化和逆DCT变换,最终恢复原始图像。该算法在图像压缩领域得到了广泛应用,如JPEG图像压缩标准就采用了DCT压缩算法。
相关问题
基于离散余弦变换的图像压缩 c语言算法实现
以下是一个基于离散余弦变换的图像压缩的C语言实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define BLOCK_SIZE 8
#define IMAGE_SIZE 256
double C[BLOCK_SIZE][BLOCK_SIZE];
double C_t[BLOCK_SIZE][BLOCK_SIZE];
/* 初始化DCT系数矩阵 */
void init_DCT_matrix()
{
for(int i=0; i<BLOCK_SIZE; i++)
{
for(int j=0; j<BLOCK_SIZE; j++)
{
if(i == 0)
{
C[i][j] = sqrt(1.0/BLOCK_SIZE);
}
else
{
C[i][j] = sqrt(2.0/BLOCK_SIZE) * cos((2*j+1)*i*M_PI/(2.0*BLOCK_SIZE));
}
C_t[j][i] = C[i][j];
}
}
}
/* 对一个8x8的图像块进行DCT变换 */
void DCT(double img[BLOCK_SIZE][BLOCK_SIZE], double dct[BLOCK_SIZE][BLOCK_SIZE])
{
double temp[BLOCK_SIZE][BLOCK_SIZE] = {0};
double temp2[BLOCK_SIZE][BLOCK_SIZE] = {0};
/* 对行进行DCT变换 */
for(int i=0; i<BLOCK_SIZE; i++)
{
for(int j=0; j<BLOCK_SIZE; j++)
{
double sum = 0;
for(int k=0; k<BLOCK_SIZE; k++)
{
sum += img[i][k] * C[j][k];
}
temp[i][j] = sum;
}
}
/* 对列进行DCT变换 */
for(int i=0; i<BLOCK_SIZE; i++)
{
for(int j=0; j<BLOCK_SIZE; j++)
{
double sum = 0;
for(int k=0; k<BLOCK_SIZE; k++)
{
sum += temp[k][i] * C_t[j][k];
}
temp2[i][j] = sum;
}
}
/* 将DCT系数矩阵赋值给输出矩阵 */
for(int i=0; i<BLOCK_SIZE; i++)
{
for(int j=0; j<BLOCK_SIZE; j++)
{
dct[i][j] = temp2[i][j];
}
}
}
/* 对一个8x8的DCT系数矩阵进行逆DCT变换 */
void IDCT(double dct[BLOCK_SIZE][BLOCK_SIZE], double img[BLOCK_SIZE][BLOCK_SIZE])
{
double temp[BLOCK_SIZE][BLOCK_SIZE] = {0};
double temp2[BLOCK_SIZE][BLOCK_SIZE] = {0};
/* 对行进行逆DCT变换 */
for(int i=0; i<BLOCK_SIZE; i++)
{
for(int j=0; j<BLOCK_SIZE; j++)
{
double sum = 0;
for(int k=0; k<BLOCK_SIZE; k++)
{
sum += dct[i][k] * C_t[k][j];
}
temp[i][j] = sum;
}
}
/* 对列进行逆DCT变换 */
for(int i=0; i<BLOCK_SIZE; i++)
{
for(int j=0; j<BLOCK_SIZE; j++)
{
double sum = 0;
for(int k=0; k<BLOCK_SIZE; k++)
{
sum += C[k][i] * temp[k][j];
}
temp2[i][j] = sum;
}
}
/* 将逆DCT变换后的像素矩阵赋值给输出矩阵 */
for(int i=0; i<BLOCK_SIZE; i++)
{
for(int j=0; j<BLOCK_SIZE; j++)
{
img[i][j] = temp2[i][j];
}
}
}
/* 对整幅图像进行DCT变换 */
void compress_image(double img[IMAGE_SIZE][IMAGE_SIZE], double dct[IMAGE_SIZE][IMAGE_SIZE])
{
init_DCT_matrix();
/* 对每个8x8的图像块进行DCT变换 */
for(int i=0; i<IMAGE_SIZE; i+=BLOCK_SIZE)
{
for(int j=0; j<IMAGE_SIZE; j+=BLOCK_SIZE)
{
double block[BLOCK_SIZE][BLOCK_SIZE] = {0};
double dct_block[BLOCK_SIZE][BLOCK_SIZE] = {0};
/* 将图像块赋值给一个8x8的矩阵 */
for(int x=0; x<BLOCK_SIZE; x++)
{
for(int y=0; y<BLOCK_SIZE; y++)
{
block[x][y] = img[i+x][j+y];
}
}
/* 对图像块进行DCT变换 */
DCT(block, dct_block);
/* 将DCT系数矩阵赋值给输出矩阵 */
for(int x=0; x<BLOCK_SIZE; x++)
{
for(int y=0; y<BLOCK_SIZE; y++)
{
dct[i+x][j+y] = dct_block[x][y];
}
}
}
}
}
/* 对整幅图像进行逆DCT变换 */
void decompress_image(double dct[IMAGE_SIZE][IMAGE_SIZE], double img[IMAGE_SIZE][IMAGE_SIZE])
{
init_DCT_matrix();
/* 对每个8x8的DCT系数矩阵进行逆DCT变换 */
for(int i=0; i<IMAGE_SIZE; i+=BLOCK_SIZE)
{
for(int j=0; j<IMAGE_SIZE; j+=BLOCK_SIZE)
{
double block[BLOCK_SIZE][BLOCK_SIZE] = {0};
double img_block[BLOCK_SIZE][BLOCK_SIZE] = {0};
/* 将DCT系数矩阵赋值给一个8x8的矩阵 */
for(int x=0; x<BLOCK_SIZE; x++)
{
for(int y=0; y<BLOCK_SIZE; y++)
{
block[x][y] = dct[i+x][j+y];
}
}
/* 对DCT系数矩阵进行逆DCT变换 */
IDCT(block, img_block);
/* 将逆DCT变换后的像素矩阵赋值给输出矩阵 */
for(int x=0; x<BLOCK_SIZE; x++)
{
for(int y=0; y<BLOCK_SIZE; y++)
{
img[i+x][j+y] = img_block[x][y];
}
}
}
}
}
int main()
{
double img[IMAGE_SIZE][IMAGE_SIZE] = {0};
double dct[IMAGE_SIZE][IMAGE_SIZE] = {0};
/* 读取图像数据 */
/* 对整幅图像进行DCT变换 */
compress_image(img, dct);
/* 对整幅图像进行逆DCT变换 */
decompress_image(dct, img);
/* 输出逆DCT变换后的图像数据 */
return 0;
}
```
以上代码实现了一个简单的基于离散余弦变换的图像压缩算法。
基于离散余弦变换的数字图像压缩算法实现c语言实现
数字图像压缩算法中,基于离散余弦变换(DCT)的压缩算法是比较常用的一种。下面是一个简单的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define BLOCK_SIZE 8 // 定义块大小为8x8
#define IMAGE_SIZE 512 // 定义图像大小为512x512
// 定义DCT系数矩阵
double c[BLOCK_SIZE][BLOCK_SIZE] = {0};
double dct_matrix[BLOCK_SIZE][BLOCK_SIZE] = {0};
void init_DCT_matrix()
{
for(int i=0;i<BLOCK_SIZE;i++){
for(int j=0;j<BLOCK_SIZE;j++){
if(i==0){
c[i][j] = sqrt(1.0/BLOCK_SIZE);
}else{
c[i][j] = sqrt(2.0/BLOCK_SIZE) * cos((2*j+1)*i*M_PI/(2*BLOCK_SIZE));
}
}
}
for(int i=0;i<BLOCK_SIZE;i++){
for(int j=0;j<BLOCK_SIZE;j++){
for(int k=0;k<BLOCK_SIZE;k++){
dct_matrix[i][j] += c[i][k] * c[j][k];
}
}
}
}
// DCT变换函数
void DCT(double block[BLOCK_SIZE][BLOCK_SIZE])
{
double tmp[BLOCK_SIZE][BLOCK_SIZE] = {0};
for(int i=0;i<BLOCK_SIZE;i++){
for(int j=0;j<BLOCK_SIZE;j++){
for(int k=0;k<BLOCK_SIZE;k++){
tmp[i][j] += c[k][i] * block[k][j];
}
}
}
for(int i=0;i<BLOCK_SIZE;i++){
for(int j=0;j<BLOCK_SIZE;j++){
block[i][j] = 0;
for(int k=0;k<BLOCK_SIZE;k++){
block[i][j] += tmp[i][k] * c[k][j];
}
}
}
}
// 块处理函数
void process_block(double block[BLOCK_SIZE][BLOCK_SIZE])
{
// 对块进行DCT变换
DCT(block);
// 对DCT系数进行量化
int quantization_table[BLOCK_SIZE][BLOCK_SIZE] = {
{16, 11, 10, 16, 24, 40, 51, 61},
{12, 12, 14, 19, 26, 58, 60, 55},
{14, 13, 16, 24, 40, 57, 69, 56},
{14, 17, 22, 29, 51, 87, 80, 62},
{18, 22, 37, 56, 68, 109, 103, 77},
{24, 35, 55, 64, 81, 104, 113, 92},
{49, 64, 78, 87, 103, 121, 120, 101},
{72, 92, 95, 98, 112, 100, 103, 99}
};
for(int i=0;i<BLOCK_SIZE;i++){
for(int j=0;j<BLOCK_SIZE;j++){
block[i][j] = round(block[i][j] / quantization_table[i][j]);
}
}
}
// 块反向处理函数
void reverse_process_block(double block[BLOCK_SIZE][BLOCK_SIZE])
{
// 对DCT系数进行反向量化
int quantization_table[BLOCK_SIZE][BLOCK_SIZE] = {
{16, 11, 10, 16, 24, 40, 51, 61},
{12, 12, 14, 19, 26, 58, 60, 55},
{14, 13, 16, 24, 40, 57, 69, 56},
{14, 17, 22, 29, 51, 87, 80, 62},
{18, 22, 37, 56, 68, 109, 103, 77},
{24, 35, 55, 64, 81, 104, 113, 92},
{49, 64, 78, 87, 103, 121, 120, 101},
{72, 92, 95, 98, 112, 100, 103, 99}
};
for(int i=0;i<BLOCK_SIZE;i++){
for(int j=0;j<BLOCK_SIZE;j++){
block[i][j] *= quantization_table[i][j];
}
}
// 对块进行IDCT变换
double tmp[BLOCK_SIZE][BLOCK_SIZE] = {0};
for(int i=0;i<BLOCK_SIZE;i++){
for(int j=0;j<BLOCK_SIZE;j++){
for(int k=0;k<BLOCK_SIZE;k++){
tmp[i][j] += c[k][i] * block[k][j];
}
}
}
for(int i=0;i<BLOCK_SIZE;i++){
for(int j=0;j<BLOCK_SIZE;j++){
block[i][j] = 0;
for(int k=0;k<BLOCK_SIZE;k++){
block[i][j] += tmp[i][k] * c[k][j];
}
block[i][j] = round(block[i][j]);
}
}
}
// 主函数
int main()
{
// 读取原始图像
FILE* fp_in = fopen("lena512.bmp", "rb");
if(fp_in == NULL){
printf("can't open file\n");
return -1;
}
unsigned char img_in[IMAGE_SIZE][IMAGE_SIZE] = {0};
fread(img_in, sizeof(unsigned char), IMAGE_SIZE*IMAGE_SIZE, fp_in);
fclose(fp_in);
// 对图像进行块处理
init_DCT_matrix();
for(int i=0;i<IMAGE_SIZE/BLOCK_SIZE;i++){
for(int j=0;j<IMAGE_SIZE/BLOCK_SIZE;j++){
double block[BLOCK_SIZE][BLOCK_SIZE] = {0};
for(int x=0;x<BLOCK_SIZE;x++){
for(int y=0;y<BLOCK_SIZE;y++){
block[x][y] = img_in[i*BLOCK_SIZE+x][j*BLOCK_SIZE+y];
}
}
process_block(block);
for(int x=0;x<BLOCK_SIZE;x++){
for(int y=0;y<BLOCK_SIZE;y++){
img_in[i*BLOCK_SIZE+x][j*BLOCK_SIZE+y] = block[x][y];
}
}
}
}
// 对处理后的图像进行反向块处理
for(int i=0;i<IMAGE_SIZE/BLOCK_SIZE;i++){
for(int j=0;j<IMAGE_SIZE/BLOCK_SIZE;j++){
double block[BLOCK_SIZE][BLOCK_SIZE] = {0};
for(int x=0;x<BLOCK_SIZE;x++){
for(int y=0;y<BLOCK_SIZE;y++){
block[x][y] = img_in[i*BLOCK_SIZE+x][j*BLOCK_SIZE+y];
}
}
reverse_process_block(block);
for(int x=0;x<BLOCK_SIZE;x++){
for(int y=0;y<BLOCK_SIZE;y++){
img_in[i*BLOCK_SIZE+x][j*BLOCK_SIZE+y] = block[x][y];
}
}
}
}
// 输出压缩后的图像
FILE* fp_out = fopen("lena512_out.bmp", "wb");
if(fp_out == NULL){
printf("can't open file\n");
return -1;
}
fwrite(img_in, sizeof(unsigned char), IMAGE_SIZE*IMAGE_SIZE, fp_out);
fclose(fp_out);
return 0;
}
```
上述代码中,我们定义了一个8x8的块大小,并使用DCT变换将每个块压缩,然后再使用反向DCT变换反向压缩,最终输出压缩后的图像。其中,量化表用于对DCT系数进行量化,可以根据具体需求设置不同的量化表。
阅读全文
相关推荐
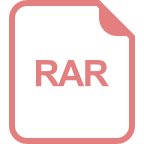
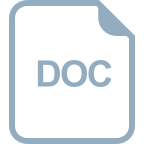
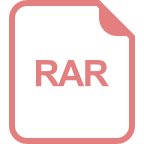
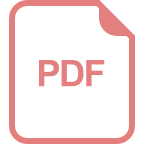
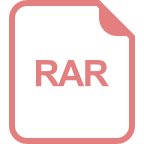
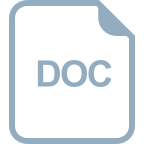
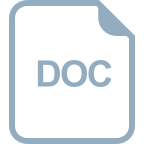
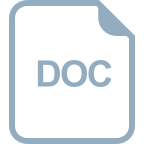
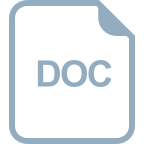
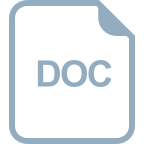
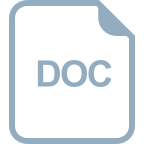
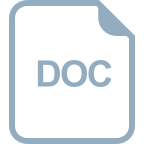

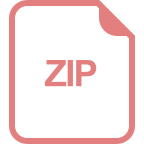