springboot +vue+elupload图片回显
时间: 2023-10-02 20:10:13 浏览: 42
在 Spring Boot 中使用 Vue.js 来上传图片并回显可以通过以下步骤来实现:
1. 在 Vue.js 中使用 el-upload 组件进行图片上传。
```
<template>
<el-upload
class="upload-demo"
action="/api/upload"
:on-success="handleSuccess"
:before-upload="beforeUpload">
<el-button size="small" type="primary">点击上传</el-button>
</el-upload>
<img :src="imageUrl" v-if="imageUrl">
</template>
<script>
export default {
data() {
return {
imageUrl: ''
}
},
methods: {
beforeUpload(file) {
const isJPG = file.type === 'image/jpeg'
const isPNG = file.type === 'image/png'
const isLt2M = file.size / 1024 / 1024 < 2
if (!isJPG && !isPNG) {
this.$message.error('只能上传 JPG/PNG 格式的图片')
return false
}
if (!isLt2M) {
this.$message.error('上传图片大小不能超过 2MB')
return false
}
return true
},
handleSuccess(res) {
this.imageUrl = res.data.url
}
}
}
</script>
```
2. 在 Spring Boot 中编写上传接口。
```
@RestController
@RequestMapping("/api")
public class UploadController {
@Value("${upload.path}")
private String uploadPath;
@PostMapping("/upload")
public Result upload(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return Result.fail("上传失败,请选择文件");
}
String fileName = file.getOriginalFilename();
String filePath = uploadPath + fileName;
File dest = new File(filePath);
try {
file.transferTo(dest);
return Result.success("上传成功", new UploadResult(fileName, filePath));
} catch (IOException e) {
e.printStackTrace();
}
return Result.fail("上传失败");
}
@Slf4j
@Data
@AllArgsConstructor
class UploadResult {
private String fileName;
private String filePath;
}
}
```
3. 在 Vue.js 中获取上传成功后的图片地址,并显示在页面中。
```
handleSuccess(res) {
this.imageUrl = res.data.url
}
```
4. 在 Spring Boot 中提供获取图片接口。
```
@GetMapping("/image/{fileName}")
public void getImage(@PathVariable("fileName") String fileName, HttpServletResponse response) {
String filePath = uploadPath + fileName;
File file = new File(filePath);
if (file.exists()) {
response.setContentType("image/jpeg");
try (FileInputStream fileInputStream = new FileInputStream(file)) {
IOUtils.copy(fileInputStream, response.getOutputStream());
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
5. 在 Vue.js 中通过图片地址来获取图片。
```
<img :src="imageUrl" v-if="imageUrl">
```
6. 最后需要在 Spring Boot 中配置上传文件路径。
```
spring:
servlet:
multipart:
max-file-size: 10MB
max-request-size: 10MB
enabled: true
resources:
static-locations: file:/path/to/upload/folder/
```
以上是上传图片并回显的实现过程,你可以根据自己的需求来进行修改和扩展。
相关推荐
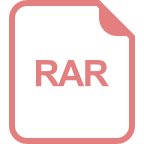
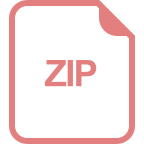
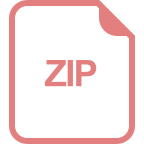














