springBoot+vue 图片上传及回显的代码实现
时间: 2023-09-08 11:08:17 浏览: 116
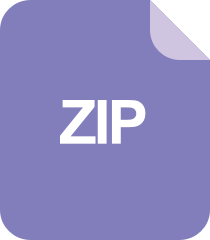
springboot+vue实现的简单人事管理系统项目源代码,java课程设计,java简单人事管理系统
后端代码实现:
1.引入相关依赖
```xml
<!-- 文件上传 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
```
2.配置文件上传相关信息
```yaml
# 文件上传限制
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
spring.servlet.multipart.enabled=true
```
3.编写文件上传接口
```java
@RestController
@RequestMapping("/api/file")
public class FileController {
@PostMapping("/upload")
public String upload(@RequestParam("file") MultipartFile file) throws IOException {
if (file.isEmpty()) {
return "文件为空";
}
String fileName = file.getOriginalFilename();
String filePath = "D:\\temp\\";
File dest = new File(filePath + fileName);
file.transferTo(dest);
return "上传成功";
}
}
```
前端代码实现:
1.安装 axios 和 element-ui
```bash
npm install axios element-ui --save
```
2.编写文件上传组件
```vue
<template>
<div>
<el-upload
class="upload-demo"
action="/api/file/upload"
:auto-upload="false"
:on-change="handleChange"
>
<el-button slot="trigger" type="primary">选取文件</el-button>
<el-button v-if="imageUrl" type="success" @click="handleUpload">上传到服务器</el-button>
<div class="el-upload__tip" slot="tip">只能上传jpg/png文件,且不超过10MB</div>
</el-upload>
<img v-if="imageUrl" :src="imageUrl" style="max-width: 100%;">
</div>
</template>
<script>
import axios from 'axios';
import { Message } from 'element-ui';
export default {
data() {
return {
imageUrl: '',
file: null,
};
},
methods: {
handleChange(file) {
this.file = file.raw;
this.imageUrl = URL.createObjectURL(this.file);
},
handleUpload() {
const formData = new FormData();
formData.append('file', this.file);
axios.post('/api/file/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data',
},
}).then(() => {
Message.success('上传成功');
}).catch(() => {
Message.error('上传失败');
});
},
},
};
</script>
```
至此,图片上传及回显的代码实现就完成了。
阅读全文
相关推荐
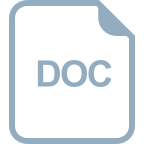
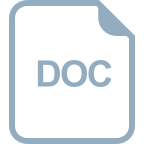





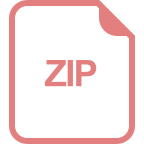

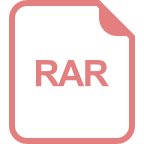
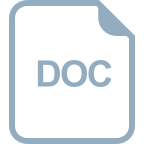




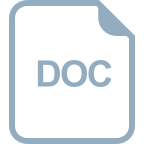
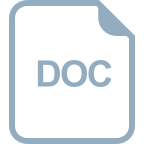