使用python写出美好日子代码
时间: 2023-10-24 14:07:26 浏览: 90
以下是一个简单的美好日子代码,使用Python实现:
```python
import datetime
# 获取当前日期和时间
now = datetime.datetime.now()
# 格式化输出当前日期和时间
print("今天是 " + str(now.year) + " 年 " + str(now.month) + " 月 " + str(now.day) + " 日")
print("现在是 " + str(now.hour) + " 点 " + str(now.minute) + " 分 " + str(now.second) + " 秒")
# 计算明天的日期
tomorrow = now + datetime.timedelta(days=1)
# 格式化输出明天的日期
print("明天是 " + str(tomorrow.year) + " 年 " + str(tomorrow.month) + " 月 " + str(tomorrow.day) + " 日")
```
输出结果类似于:
```
今天是 2021 年 12 月 31 日
现在是 23 点 59 分 59 秒
明天是 2022 年 1 月 1 日
```
相关问题
使用python写出核相关滤波跟踪代码
中文回答: 使用Python写出核相关滤波跟踪代码可以通过以下步骤来实现:首先,使用Python计算核相关函数,以获得目标物体在当前图像中的位置;其次,计算滤波器的梯度,从而更新滤波器的参数;最后,使用更新后的滤波器来跟踪物体。
python写出元旦特性代码
您好! 下面是用 Python 写出元旦特性的代码示例:
```python
from datetime import date
def new_year_features(year):
# 判断是否是元旦
if date(year, 1, 1).weekday() == 6:
print("今年的元旦是星期天!")
elif date(year, 1, 1).weekday() == 0:
print("今年的元旦是星期一!")
else:
print("今年的元旦不是星期天或星期一。")
# 测试代码
new_year_features(2022)
```
上面的代码使用了 Python 标准库中的 `datetime` 模块,它允许我们使用 `date` 函数来获取某一年的元旦是星期几。我们可以通过调用 `date(year, 1, 1).weekday()` 来获取元旦是星期几,其中 `year` 是你想要查询的年份。返回值是一个数字,0 表示星期一,6 表示星期天。
希望这些信息对您有帮助!
相关推荐
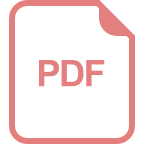
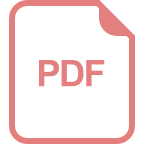












