写一个Python爬虫爬取a股数据
时间: 2023-05-19 16:05:19 浏览: 195
好的,以下是一个简单的Python爬虫代码,可以爬取A股数据:
```python
import requests
from bs4 import BeautifulSoup
url = 'http://quote.eastmoney.com/center/gridlist.html#hs_a_board'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
table = soup.find('table', {'id': 'table_wrapper-table'})
rows = table.find_all('tr')
for row in rows:
cols = row.find_all('td')
for col in cols:
print(col.text.strip(), end='\t')
print()
```
这个爬虫会爬取东方财富网的A股数据,并将其打印出来。
相关问题
写一个python爬虫爬取a股数据
可以使用 Python 的 requests 和 BeautifulSoup 库来爬取 A 股数据。以下是一个示例代码,可以获取某只股票的历史数据:
```python
import requests
from bs4 import BeautifulSoup
def get_stock_data(stock_code):
url = 'http://quotes.money.163.com/trade/lsjysj_{}.html'.format(stock_code)
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
table = soup.find_all('table')[0]
rows = table.find_all('tr')
data = []
for row in rows[1:]:
cols = row.find_all('td')
row_data = [col.text.strip() for col in cols]
data.append(row_data)
return data
```
其中,`stock_code` 是股票代码,比如 `600519` 代表茅台。该函数会返回一个二维数组,每个元素代表一行历史数据,包括日期、开盘价、收盘价、最高价、最低价、成交量和成交金额等信息。你可以根据需要对数据进行进一步处理和分析。
python爬取A股股价并绘制成折线图
首先,你需要准备一些必要的工具:
1. 安装Python:你可以从官网下载并安装最新版本的Python(https://www.python.org/downloads/)。
2. 安装爬虫库:可以使用Python的第三方库来实现网络爬虫功能,常用的爬虫库有BeautifulSoup、Scrapy、requests等。
3. 安装数据分析库:主要用于处理数据,如NumPy、Pandas等。
4. 安装可视化库:主要用于将数据可视化,如Matplotlib、Seaborn等。
然后,你就可以开始编写代码了。
首先,你需要使用爬虫库(如requests)来爬取A股股价数据,并使用数据分析库(如Pandas)来处理数据。
具体来说,你可以使用requests库发送HTTP请求,获取网页内容,然后使用Pandas库将网页内容解析为DataFrame格式,方便后续处理。
接下来,你可以使用可视化库(如Matplotlib)来绘制折线图。
具体来说,你可以使用Matplotlib库的plot方法绘制折线图,然后使用show方法显示图表。
示例代码如下:
```python
import requests
import pandas as pd
import matplotlib.pyplot as plt
# 爬取A股股价数据
response = requests.get('http://stock.example.com/stock_data.html')
html = response.text
# 使用
相关推荐
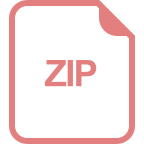
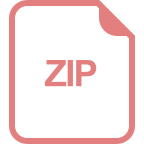













