生成一段LDA线性判别分析用numpy
时间: 2023-11-14 16:44:11 浏览: 39
import numpy as np
# 生成2类数据,每类数据有100个样本,每个样本有5个特征
mean1 = np.array([1, 2, 3, 4, 5])
mean2 = np.array([5, 4, 3, 2, 1])
cov = np.array([[1, 0, 0, 0, 0],
[0, 1, 0, 0, 0],
[0, 0, 1, 0, 0],
[0, 0, 0, 1, 0],
[0, 0, 0, 0, 1]])
X1 = np.random.multivariate_normal(mean1, cov, 100)
X2 = np.random.multivariate_normal(mean2, cov, 100)
# 将两类数据合并成一个数据集
X = np.vstack((X1, X2))
# 生成对应的标签
y1 = np.zeros(100)
y2 = np.ones(100)
y = np.hstack((y1, y2))
# 计算每个类别的均值向量
mean_vec1 = np.mean(X1, axis=0)
mean_vec2 = np.mean(X2, axis=0)
# 计算类内散度矩阵
S1 = np.zeros((5, 5))
for row in X1:
row, mean_vec1 = row.reshape(5, 1), mean_vec1.reshape(5, 1)
S1 += (row - mean_vec1).dot((row - mean_vec1).T)
S2 = np.zeros((5, 5))
for row in X2:
row, mean_vec2 = row.reshape(5, 1), mean_vec2.reshape(5, 1)
S2 += (row - mean_vec2).dot((row - mean_vec2).T)
Sw = S1 + S2
# 计算类间散度矩阵
mean_vec = np.mean(X, axis=0)
mean_vec = mean_vec.reshape(5, 1)
Sb = np.zeros((5, 5))
Sb += 100 * (mean_vec1 - mean_vec).dot((mean_vec1 - mean_vec).T)
Sb += 100 * (mean_vec2 - mean_vec).dot((mean_vec2 - mean_vec).T)
# 计算Sw的逆矩阵
Sw_inv = np.linalg.inv(Sw)
# 计算最优的投影方向
w = Sw_inv.dot(mean_vec1 - mean_vec2)
# 对数据进行投影
X_lda = X.dot(w)
# 输出投影后的数据
print(X_lda)
相关推荐
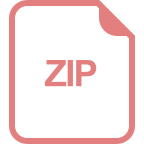














