编写程序,使用fork()产生一个子进程。在父进程和子进程中都打开一个共同的 文件,该文件中含有一个数字。父子进程分别读取文件中的数字到各自内存变量 中,对变量的值进行+1操作后重新写回文件中。父子进程反复“执行读取文件到 内存变量->将变量的值+1->重新写回文件”这一组操作固定次数,最后读取文件 中的数字到终端屏幕上显示。
时间: 2024-03-25 22:38:36 浏览: 11
下面是一个实现上述功能的简单程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/wait.h>
#define FILENAME "test.txt" // 共享文件名
#define MAX_COUNT 1000 // 操作次数
int main() {
int fd;
pid_t pid;
int num = 0;
// 打开共享文件,如果不存在则创建
fd = open(FILENAME, O_RDWR | O_CREAT, 0666);
if (fd < 0) {
perror("open");
exit(1);
}
// 初始时向文件中写入数字 0
if (write(fd, &num, sizeof(num)) != sizeof(num)) {
perror("write");
exit(1);
}
// 创建子进程
pid = fork();
if (pid < 0) {
perror("fork");
exit(1);
}
// 父进程和子进程都执行以下代码
for (int i = 0; i < MAX_COUNT; i++) {
// 将文件读入内存变量
if (lseek(fd, 0, SEEK_SET) < 0) {
perror("lseek");
exit(1);
}
if (read(fd, &num, sizeof(num)) != sizeof(num)) {
perror("read");
exit(1);
}
// 将内存变量加 1
num++;
// 将内存变量写回文件
if (lseek(fd, 0, SEEK_SET) < 0) {
perror("lseek");
exit(1);
}
if (write(fd, &num, sizeof(num)) != sizeof(num)) {
perror("write");
exit(1);
}
}
// 等待子进程结束
if (pid > 0) {
wait(NULL);
}
// 将文件读入内存变量并输出到终端屏幕
if (lseek(fd, 0, SEEK_SET) < 0) {
perror("lseek");
exit(1);
}
if (read(fd, &num, sizeof(num)) != sizeof(num)) {
perror("read");
exit(1);
}
printf("The number is: %d\n", num);
// 关闭文件并删除
close(fd);
unlink(FILENAME);
return 0;
}
```
该程序首先打开一个共享文件,然后创建子进程。父进程和子进程都执行一个循环,每次循环将文件读入内存变量、对变量加 1、将变量写回文件。最后父进程等待子进程结束,然后将文件读入内存变量并输出到终端屏幕上。最后关闭文件并删除。
需要注意的是,对文件的读写操作要使用 lseek() 函数先将文件指针移动到文件开头,否则读写操作可能会失败。另外,要使用 O_RDWR 标志打开文件,以便读写都能进行。
相关推荐
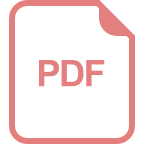
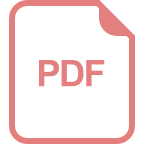














