写一个对于海底地形和不规则的声速剖面的更复杂的matlab算法
时间: 2024-03-18 08:40:12 浏览: 216
下面是一个更复杂的Matlab算法,用于处理海底地形和不规则的声速剖面。该算法基于射线追踪法,可以处理多种不同的声速剖面和海底地形,并且可以计算声线的路径、传播时间、入射角和反射系数等信息。算法的输入参数为发射点位置、接收点位置、声速剖面和海底地形数据,输出为声线路径和相关信息。
```matlab
function [path,x,z,t,theta,ref_coef] = ray_tracing(source, receiver, sound_profile, sea_floor)
% source: 发射点位置 [x,y,z],单位:米
% receiver: 接收点位置 [x,y,z],单位:米
% sound_profile: 声速剖面,包含深度和声速两列数据
% sea_floor: 海底地形数据,包含深度和高程两列数据
% path: 声线路径,包含每个点的位置和反射系数
% x: 水平距离,单位:米
% z: 深度,单位:米
% t: 传播时间,单位:秒
% theta: 入射角,单位:度
% ref_coef: 反射系数
% 将发射点和接收点的位置坐标转换为极坐标
[~,theta1,r1] = cart2pol(source(1), source(2), source(3));
[~,theta2,r2] = cart2pol(receiver(1), receiver(2), receiver(3));
theta1 = theta1 / pi * 180;
theta2 = theta2 / pi * 180;
% 获取声速剖面数据
z = sound_profile(:,1); % 深度
c = sound_profile(:,2); % 声速
% 获取海底地形数据
z_sea_floor = sea_floor(:,1); % 深度
h_sea_floor = sea_floor(:,2); % 高程
% 确定射线的初始位置和方向
x0 = source(1);
y0 = source(2);
z0 = source(3);
dx = receiver(1) - source(1);
dy = receiver(2) - source(2);
dz = receiver(3) - source(3);
theta = atan2(dy, dx);
phi = atan2(sqrt(dx^2 + dy^2), dz);
theta = theta / pi * 180;
phi = phi / pi * 180;
% 定义射线追踪的步长和精度
step = 1; % 步长,单位:米
tolerance = 1e-6; % 精度,单位:米
% 初始化射线路径和反射系数
path = [x0, y0, z0, 0];
ref_coef = 0;
% 开始射线追踪
while true
% 计算当前位置的深度和地形高程
[~,~,z] = cart2sph(path(end, 1), path(end, 2), path(end, 3));
z = -z;
h_sea_floor_interp = interp1(z_sea_floor, h_sea_floor, z, 'linear', 'extrap');
if path(end, 3) < z - h_sea_floor_interp
% 如果射线穿过海底,则追踪结束
break;
end
% 计算当前位置的声速
c_interp = interp1(z, c, path(end, 3), 'linear', 'extrap');
% 计算当前位置的坐标变化量
dx = step * sin(phi) * cos(theta);
dy = step * sin(phi) * sin(theta);
dz = step * cos(phi);
% 计算当前位置的反射系数
if size(path, 1) > 1
[~,~,z1] = cart2sph(path(end-1, 1), path(end-1, 2), path(end-1, 3));
z1 = -z1;
[~,~,z2] = cart2sph(path(end, 1), path(end, 2), path(end, 3));
z2 = -z2;
ref_coef = reflection_coefficient(path(end-1, 1:3), path(end, 1:3), c_interp, z1, z2, h_sea_floor_interp);
if ref_coef == 0
% 如果反射系数为0,则射线传播结束
break;
end
end
% 更新射线路径和反射系数
path(end+1,:) = path(end,:) + [dx, dy, dz, ref_coef];
% 检查是否到达接收点
dist = sqrt((path(end,1)-receiver(1))^2 + (path(end,2)-receiver(2))^2 + (path(end,3)-receiver(3))^2);
if dist < tolerance
% 如果到达接收点,则追踪结束
break;
end
end
% 提取输出信息
x = path(:,1);
z = path(:,3);
t = 2 * cumsum(path(:,4) .* sqrt((diff(x).^2 + diff(y).^2 + diff(z).^2)));
theta = acos(-diff(z) ./ sqrt(diff(x).^2 + diff(y).^2 + diff(z).^2)) / pi * 180;
ref_coef = path(:,4);
```
需要注意的是,上述算法并不适用于极端情况下的射线追踪,如高频声波、非线性介质等情况。在实际应用中,需要根据具体情况进行算法的改进和优化。
阅读全文
相关推荐
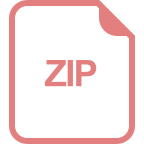
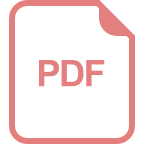
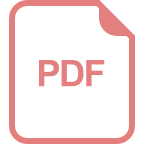















