canvas moveTo和lineTo绘制多条路径循环绑定事件
时间: 2023-07-31 10:08:06 浏览: 46
要绑定循环绘制的多个路径的事件,您可以将路径的坐标存储在数组中,然后使用循环为每个路径添加事件监听器。
下面是一个简单的示例代码:
```javascript
// 创建画布
let canvas = document.createElement('canvas');
canvas.width = 500;
canvas.height = 500;
document.body.appendChild(canvas);
let ctx = canvas.getContext('2d');
// 定义路径坐标数组
let paths = [
{ points: [{ x: 50, y: 50 }, { x: 100, y: 100 }, { x: 50, y: 150 }], color: 'red' },
{ points: [{ x: 150, y: 50 }, { x: 200, y: 100 }, { x: 150, y: 150 }], color: 'blue' }
];
// 循环绘制路径和绑定事件
for (let i = 0; i < paths.length; i++) {
let path = paths[i];
// 绘制路径
ctx.beginPath();
ctx.moveTo(path.points[0].x, path.points[0].y);
for (let j = 1; j < path.points.length; j++) {
ctx.lineTo(path.points[j].x, path.points[j].y);
}
ctx.strokeStyle = path.color;
ctx.stroke();
// 绑定事件
canvas.addEventListener('click', function(event) {
let x = event.offsetX;
let y = event.offsetY;
// 检查事件是否发生在当前路径上
ctx.beginPath();
ctx.moveTo(path.points[0].x, path.points[0].y);
for (let j = 1; j < path.points.length; j++) {
ctx.lineTo(path.points[j].x, path.points[j].y);
}
if (ctx.isPointInPath(x, y)) {
alert('You clicked on path ' + (i + 1) + '!');
}
});
}
```
在上面的示例中,我们定义了一个路径坐标数组 `paths`,其中包含两个路径的坐标和颜色信息。我们使用循环绘制了两条路径,并为每个路径添加了点击事件监听器。在事件监听器中,我们检查事件是否发生在当前路径上,并在点击事件发生时执行相应的操作。
注意,为了避免重复添加事件监听器,我们将事件监听器放在循环外部,而不是在循环内部。
相关推荐
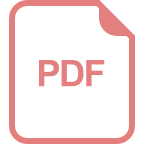
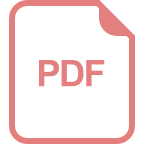
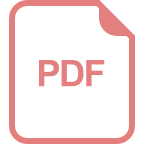














